Introduction:
Top Interview Questions On OOPs Concept In Java

1.What is object-oriented programming?
Ans: Object-oriented programming (OOP) is a programming paradigm that is based on the concept of objects, which can contain data and code to manipulate that data.
In OOP, the focus is on objects rather than functions, procedures or logic. Objects can be thought of as real-world entities, such as a person, car, or bank account, which have attributes (data) and behaviors (methods).
2. What are the four fundamental principles of object-oriented programming?
Ans: The four fundamental principles of object-oriented programming (OOP) are:
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
3. What is inheritance?
Ans: Inheritance is a concept in object-oriented programming that allows a new class to be based on an existing class, inheriting its attributes and methods. The existing class is called the parent or superclass, and the new class is called the child or subclass.
For example, suppose you have a class called Animal that has attributes like name, age, and species, and methods like eat(), sleep(), and move(). You can create a subclass of Animal called Dog that inherits all the attributes and methods of Animal, but also has its own attributes and methods like breed and bark(). The Dog class can override or add new behavior to the methods inherited from Animal, but it also retains the behavior of Animal.
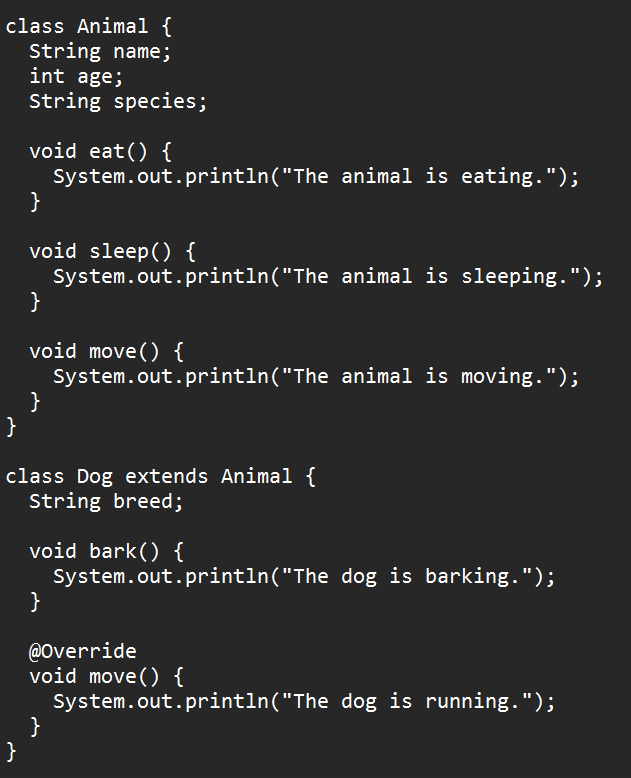
4. What is polymorphism?
Ans: Polymorphism is a concept in object-oriented programming that allows objects of different classes to be treated as if they were objects of the same class.
Here’s a simple example to illustrate polymorphism in Java:

5.What is encapsulation?
Ans: Encapsulation is the process of hiding the internal details of an object from the outside world and providing a public interface to interact with the object.
6. What is abstraction?
Ans: Abstraction is the process of focusing on essential features of an object and ignoring the non-essential details.
In other words, abstraction provides a way to represent complex objects by hiding their complexity and presenting only the necessary information to the user.
7. What is a class?
Ans : In Java, a class is a blueprint or a template for creating objects. It defines a set of properties (data members) and methods (functions) that an object of that class can have.
8. What is the difference between a static method and an instance method?
Ans: In simple terms, a static method belongs to the class itself, while an instance method belongs to an instance of the class.
Here are some key differences:
- Accessing the method: To call a static method, you use the class name followed by the method name (e.g. ClassName.staticMethod()). To call an instance method, you use an instance of the class followed by the method name (e.g. obj.instanceMethod()).
- Memory allocation: Static methods are loaded into memory when the class is loaded, while instance methods are loaded into memory when an object of the class is created.
- Use of instance variables: Static methods can only access static variables and cannot access instance variables. Instance methods can access both static and instance variables.
- Overriding: Static methods cannot be overridden, while instance methods can be overridden in a subclass.
9. What is a final class, method, and variable?
Ans: In Java, a final class, method, or variable is one that cannot be changed once it is initialized.
- Final Class: A final class is a class that cannot be subclassed. This means that the methods and variables in the class cannot be overridden or modified in any way by a subclass. To create a final class, you simply add the keyword “final” before the class declaration, like this:
final class MyFinalClass {...}
- Final Method: A final method is a method that cannot be overridden by a subclass. This means that the subclass cannot change the implementation of the method. To create a final method, you simply add the keyword “final” before the method declaration, like this:
public final void myFinalMethod(){...}
- Final Variable: A final variable is a variable that cannot be changed after it is initialized. This means that the value of the variable cannot be modified once it is set. To create a final variable, you simply add the keyword “final” before the variable declaration, like this:
final int MY_FINAL_VARIABLE = 10;
10. What is the purpose of the “this” keyword?
There can be a lot of usage of Java this keyword. In Java, this is a reference variable that refers to the current object.
Reference:
Read More Blogs: