Introduction:
Welcome to our Top Data Structure Interview Questions for the website! This interview process is designed to evaluate candidates’ proficiency in fundamental data structure concepts, algorithmic problem-solving skills, and their ability to write efficient and maintainable code.
As we strive to build a dynamic and innovative team, assessing candidates in these areas is crucial for ensuring that they possess the technical acumen needed to contribute to the success of our website.
In this Blog, you are going to read more about Top Data Structure Interview Questions.
Top Data Structure Interview Questions
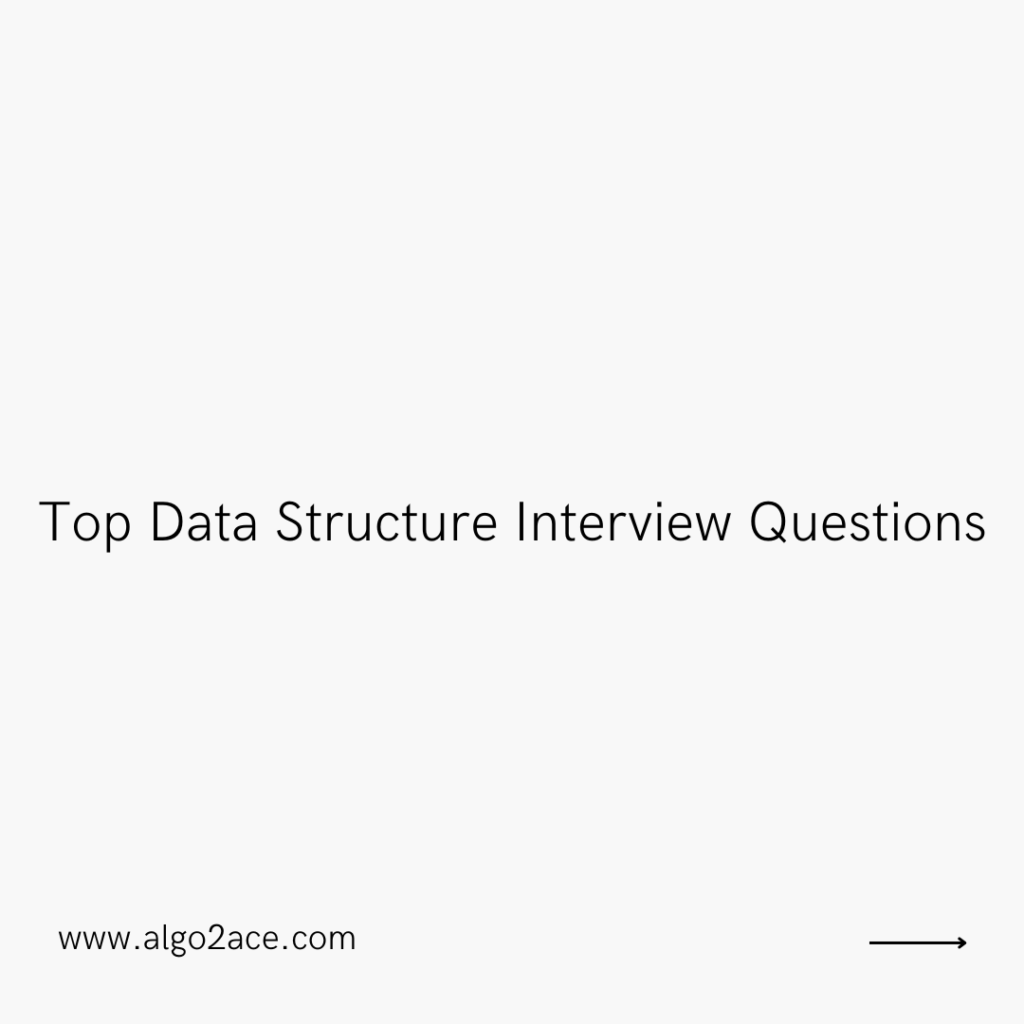
1. What is a data structure?
A data structure is a way of organizing and storing data in a computer’s memory or disk storage in a particular way that enables efficient access, modification, and retrieval of that data.
It provides a framework for organizing data that is easier to understand, manage, and manipulate. Data structures can be used to store and manipulate different types of data, including integers, characters, floating-point numbers, and more complex data types such as lists, trees, graphs, and maps.
2. What is the difference between an array and a linked list?
An array is a data structure that stores a collection of elements of the same data type in contiguous memory locations. The elements of an array are accessed using an index, which represents the position of the element in the array. The size of an array is fixed and declared at the time of creation.
On the other hand, a linked list is a data structure that consists of a collection of nodes, where each node contains a value and a pointer to the next node in the list. The nodes of a linked list are not stored in contiguous memory locations and are connected via pointers, allowing them to be inserted and removed at any position in the list. The size of a linked list is dynamic and can grow or shrink as needed.
3. What is a stack and a queue, and what are their applications?
A stack and a queue are two fundamental abstract data types in computer science that allow the storage and retrieval of data. They are used in a variety of applications, including algorithms, programming languages, operating systems, and many more.
Some applications of stacks include:
Function calls and recursive algorithms, where each function call is added to the stack, and the last-called function is executed first.
Expression evaluation, where operators and operands are added to the stack and evaluated in a specific order.
Undo/redo operations in text editors, where each change is added to the stack, and the last change can be undone or redone by popping or pushing the stack.
4. What is the difference between a binary search tree and a hash table?
A binary search tree (BST) and a hash table are two different data structures used for storing and retrieving data in computer science.
The main differences between a binary search tree and a hash table are:
Search time: Binary search trees have a time complexity of O(log n) on average, which is slower than the average time complexity of O(1) for hash tables. However, binary search trees have a more predictable worst-case time complexity of O(n), while hash tables can have a worst-case complexity of O(n) if there are many collisions.
Insertion and deletion: Binary search trees have a time complexity of O(log n) for insertion and deletion, while hash tables have a time complexity of O(1) on average. However, hash tables may need to be rehashed and resized to maintain performance, which can be a more expensive operation.
Memory usage: Binary search trees require more memory than hash tables, as they store pointers to child nodes in addition to the data. In contrast, hash tables only store the key-value pairs and the array indices.
Ordering: Binary search trees maintain the order of the elements based on their values, while hash tables do not maintain any specific order.
5. What is a sorting algorithm, and what are some common types?
A sorting algorithm is a set of instructions that take an unordered list of elements and arrange them in a specific order, such as ascending or descending order.
There are many different sorting algorithms, but some of the most common types include:
Bubble Sort: Bubble sort compares adjacent elements in the list and swaps them if they are in the wrong order. This process is repeated until the list is fully sorted. Bubble sort has a time complexity of O(n^2) and is often used for educational purposes due to its simplicity.
Selection Sort: The selection sort selects the smallest element in the list and places it at the beginning of the list. This process is repeated for the remaining unsorted portion of the list until the entire list is sorted. Selection sort also has a time complexity of O(n^2).
Insertion Sort: Insertion sort iterates over the list and inserts each element into its proper sorted position in the list. This process is repeated until the entire list is sorted. Insertion sort has a time complexity of O(n^2), but its average case and best-case complexity can be improved to O(n) when the list is nearly sorted.
Merge Sort: Merge sort uses a divide-and-conquer approach to sort the list. The list is divided into smaller sublists, which are recursively sorted and merged until the entire list is sorted. Merge sort has a time complexity of O(n log n) and is a stable sorting algorithm (meaning it preserves the relative order of equal elements).
Quick Sort: Quick sort is also a divide-and-conquer algorithm that selects a pivot element and partitions the list into elements smaller than the pivot and elements greater than the pivot. The algorithm then recursively sorts each partition until the entire list is sorted. Quick sort has an average time complexity of O(n log n) but can have a worst-case complexity of O(n^2) if the pivot element is not well chosen.
6. What is recursion, and how is it used in data structures?
Recursion is a programming technique that involves a function calling itself. In other words, a function is designed to solve a problem by calling itself with smaller versions of the same problem until the problem can be solved trivially.
Recursion is often used in data structures to simplify the implementation of certain algorithms. For example, recursive functions are commonly used to traverse tree-based data structures, such as binary search trees, where each node has two child nodes. A recursive function can be used to traverse the left and right child nodes of each node until the desired node is found.
7. How do you implement a linked list in code? in Java
Here’s an example of a singly linked list:
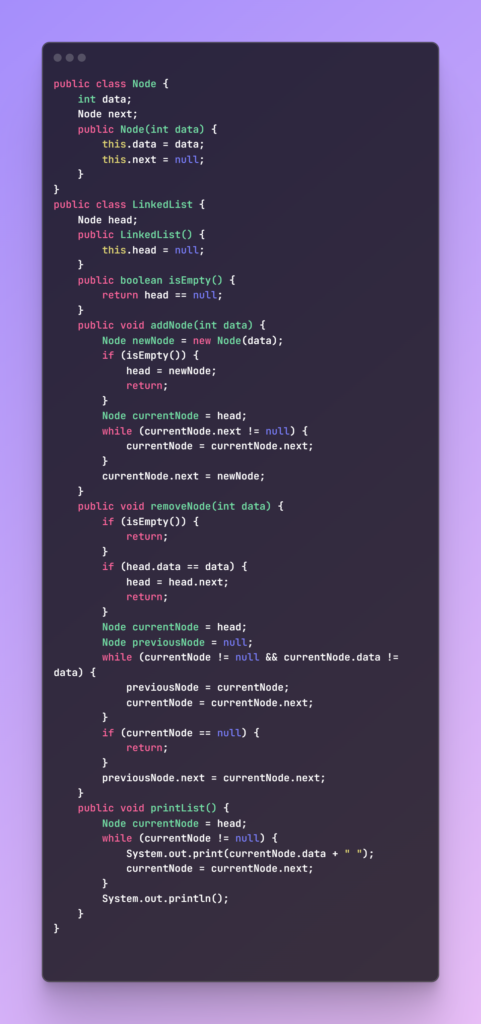
this implementation, each node in the linked list is represented by a Node class that contains an int data field and a Node reference to the next node in the list. The LinkedList class has a head field pointing to the first node in the list.
The isEmpty() method checks if the list is empty.
The addNode() method adds a new node to the end of the list by traversing to the last node and setting its next reference to the new node. The removeNode() method removes the first node with the specified data by traversing the list and updating the next reference of the previous node to skip over the current node.
The printList() method traverses the list and prints the data of each node.
8. What is a priority queue, and how is it different from a regular queue?
A priority queue is a data structure in which each element has a priority value associated with it. Elements with higher priority are dequeued before elements with lower priority.
A priority queue is different from a regular queue because, in a regular queue, elements are dequeued in the order they are enqueued, whereas in a priority queue, elements with higher priority are dequeued before elements with lower priority.
In a priority queue, the element with the highest priority is always at the front of the queue and is the first to be dequeued. When two elements have the same priority, the order of dequeuing depends on the specific implementation of the priority queue.
Some priority queues maintain a first-in-first-out (FIFO) ordering for elements with the same priority, while others may use additional criteria, such as the order in which elements were inserted or their unique identifiers, to break ties.
9. What is dynamic programming, and how is it related to data structures?
Dynamic programming is a technique for solving complex problems by breaking them down into smaller subproblems and solving each subproblem only once, then storing the results in a table or memory to avoid redundant computations. The stored results can be used to solve larger subproblems, eventually leading to the solution of the original problem.
Dynamic programming is related to data structures because it often uses data structures to store the results of subproblems. The choice of data structure depends on the specific problem being solved and the operations required for storing and accessing the results.
For example, if the problem involves finding the longest common subsequence of two strings, a dynamic programming algorithm can be used to build a matrix to store the lengths of all common subsequences of the substrings of the two strings.
The matrix can be built iteratively by comparing characters in the two strings and using the results of smaller subproblems to solve larger subproblems. The matrix is then used to construct the longest common subsequence by backtracking through the matrix from the bottom right corner.
The choice of data structure in this case is a two-dimensional array to store the lengths of common subsequences. Other problems may require different data structures, such as a hash table, a tree, or a priority queue.
10. What is a graph, and how do you represent it in code?
A graph is a data structure that consists of a set of vertices (also known as nodes) and a set of edges that connect pairs of vertices. Graphs can be used to model a wide range of problems, including network topologies, social networks, transportation systems, and many others.
In code, a graph can be represented using various data structures, including adjacency lists, adjacency matrices, and edge lists.
An adjacency list is a common way to represent a graph in code, where a list of its neighboring vertices represents each vertex. For example, in Java, we can represent an undirected graph using an adjacency list as follows:
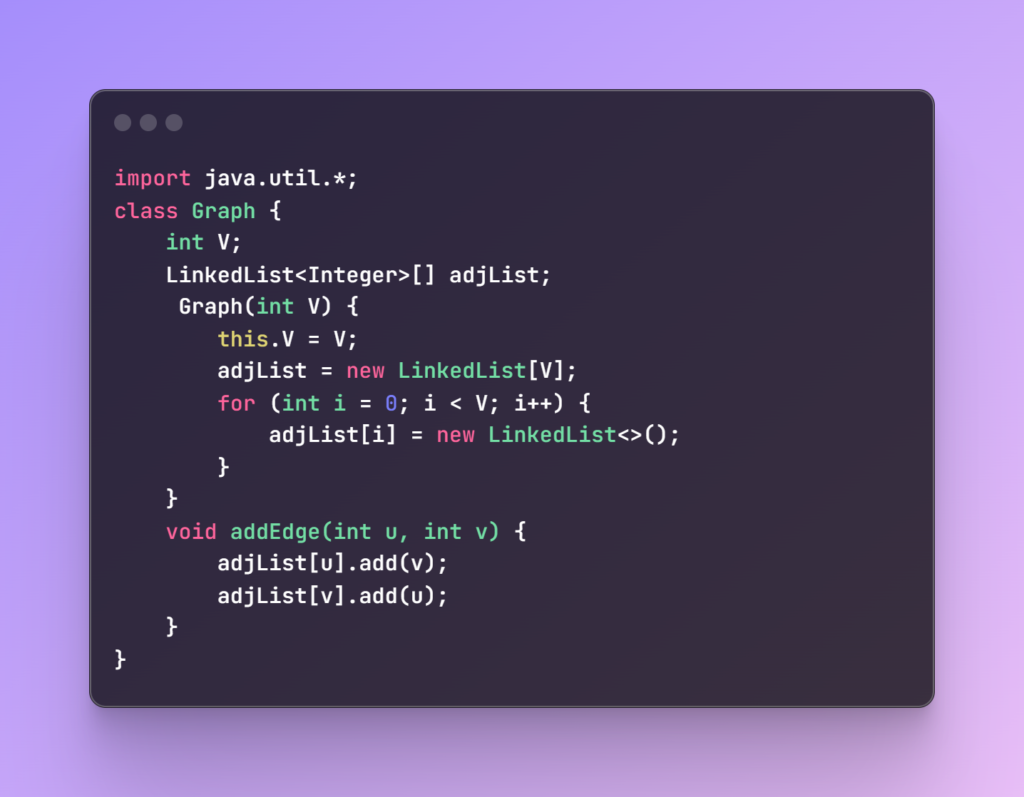
In this implementation, V represents the number of vertices in the graph, and adjList is an array of linked lists, where each linked list represents the neighbors of a vertex. The addEdge method adds an undirected edge between vertices u and v by adding v to the linked list of u and vice versa.
Another way to represent a graph in code is using an adjacency matrix, where a matrix of size V x V is used to represent the edges between vertices. Each element in the matrix represents whether there is an edge between two vertices or not.
An edge list is another way to represent a graph in code, where each element in the list represents an edge between two vertices. Each element can be represented as a tuple (u, v) representing an edge between vertices u and v. This representation can be useful when the graph is sparse, meaning that there are relatively few edges compared to the number of vertices.
Reference :
Read More Blogs:
String Related Interview Questions In Java
Top Amazon Data Structure Interview Questions
Conclusion:
In conclusion, Top Data Structure Interview Questions interview for our website is designed to assess candidates’ proficiency in fundamental concepts and their problem-solving abilities.
Throughout the interview process, candidates were evaluated on their understanding of key data structures such as arrays, linked lists, trees, and graphs, as well as their ability to apply these structures to solve real-world problems.