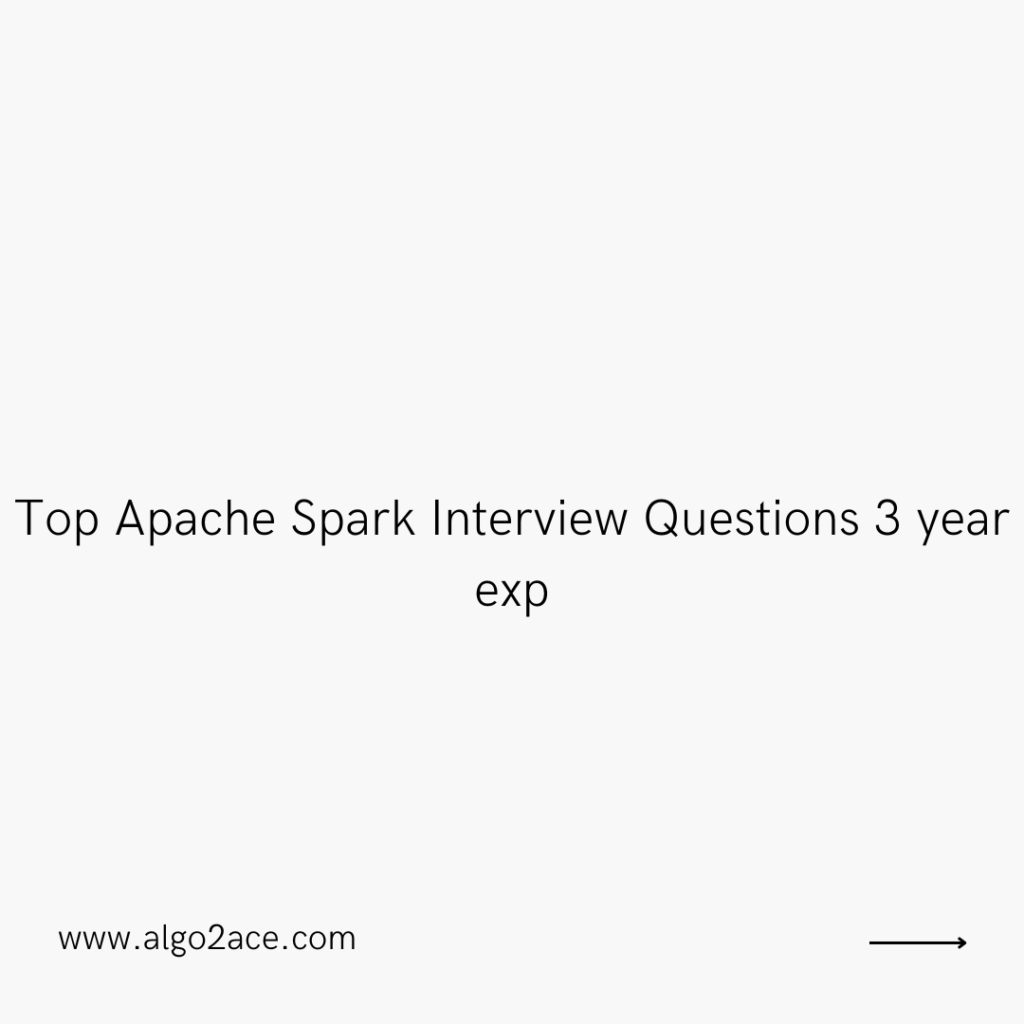
1.Describe the architecture of Apache Spark.
Ans: The architecture of Apache Spark is designed to handle large-scale data processing and analytics tasks efficiently. It consists of various components that work together to execute Spark applications. Here’s a high-level overview of the architecture:
- Cluster Manager:
- Apache Spark can integrate with different cluster managers like Apache Mesos, Hadoop YARN, or it can run in standalone mode.
- The cluster manager is responsible for allocating resources (CPU, memory) and managing the execution of Spark applications on a cluster of machines.
- Driver Program:
- The driver program is the entry point of a Spark application.
- It runs the main function and creates a SparkContext, which is the central coordinator of Spark.
- The driver program defines the Spark application, including the transformations and actions to be performed on the data.
- SparkContext:
- SparkContext (sc) is responsible for establishing a connection with the cluster manager and coordinating the execution of Spark tasks.
- It represents the entry point for interacting with Spark functionalities.
- SparkContext coordinates the distribution of tasks across the worker nodes, manages the execution environment, and handles fault tolerance.
- Executors:
- Executors are worker nodes in the Spark cluster that execute tasks assigned to them by the driver program.
- Each executor runs multiple concurrent tasks in separate threads and manages the data and computations for those tasks.
- Executors are responsible for caching and storing data in memory or disk, as directed by the application.
- Cluster Manager Scheduler:
- The cluster manager scheduler is responsible for managing the allocation of resources (CPU, memory) to the Spark application.
- It works closely with the cluster manager to acquire and release resources based on the application’s requirements.
- Resilient Distributed Datasets (RDD):
- RDD is the fundamental data structure in Spark. It represents an immutable, distributed collection of objects across the cluster.
- RDDs can be created from data stored in Hadoop Distributed File System (HDFS), local file systems, or by transforming existing RDDs.
- RDDs support both parallel processing and fault tolerance by dividing the data into partitions that can be processed in parallel across the cluster.
- Data Sources and Sinks:
- Spark can read data from various sources like HDFS, HBase, Hive, Kafka, etc.
- It can also write data to multiple sinks like HDFS, databases, and file systems.
- Spark provides a unified API to interact with different data sources and sinks, making it easy to read, process, and write data.
2. What is lazy evaluation in Spark? How does it help in optimizing performance?
Ans: Lazy evaluation is a key feature in Apache Spark that refers to the postponement of computation until it is absolutely necessary.
Here’s how lazy evaluation works and how it helps optimize performance in Spark:
- Transformations:
- When you perform transformations on RDDs or DataFrames in Spark, such as filter(), map(), or groupBy(), Spark doesn’t immediately execute these transformations.
- Instead, Spark builds a logical execution plan, called a Directed Acyclic Graph (DAG), which represents the sequence of transformations to be applied.
- Spark records the lineage information, which is the history of the transformations applied to the base data. This lineage information enables fault tolerance and data recovery.
- Actions:
- Actions in Spark, such as count(), collect(), or saveAsTextFile(), trigger the execution of the DAG by initiating the actual computations on the RDDs.
- At the point of the action, Spark optimizes the execution plan based on the transformations applied and performs the necessary computations on the data.
- Benefits of Lazy Evaluation and Performance Optimization:
- Pipeline Fusion:
- Spark combines multiple transformations into a single operation, known as pipeline fusion or pipelining.
- This optimization reduces the amount of data shuffled between stages, minimizing the disk I/O and network overhead.
- By fusing transformations together, Spark can perform operations more efficiently and minimize the overall computation time.
- Optimized Execution Plan:
- Spark’s Catalyst optimizer analyzes the entire DAG and applies various optimization techniques, such as predicate pushdown, column pruning, and code generation, to generate an optimized execution plan.
- By analyzing the DAG as a whole, Spark can eliminate unnecessary computations, apply predicate filters early in the pipeline, and generate optimized bytecode for faster execution.
- Reduced Disk and Memory Usage:
- Lazy evaluation allows Spark to determine the minimal set of data required for executing the actions.
- This minimizes the need for intermediate results to be written to disk, reducing disk I/O operations and saving memory usage.
- Spark can keep most of the intermediate data in memory, which significantly improves performance by avoiding costly disk read/write operations.
- Dynamic Optimization:
- Spark’s lazy evaluation enables dynamic optimization by allowing the framework to adapt its execution plan based on runtime statistics and conditions.
- This dynamic optimization includes partition pruning, data skew handling, and adaptive query execution, among others, to enhance performance based on the actual data and workload characteristics.
- Pipeline Fusion:
3. What are the different types of joins available in Spark? Provide examples.
Ans: In Spark, there are several types of joins available to combine data from different DataFrames or RDDs. Here are the commonly used join types in Spark:
- Inner Join:
- Inner join returns only the matching records from both DataFrames based on a common key.
- Example:
df1.join(df2, "commonKey")
- Left Outer Join:
- Left outer join returns all the records from the left DataFrame and the matching records from the right DataFrame.
- Example:
df1.join(df2, "commonKey", "left_outer")
- Right Outer Join:
- Right outer join returns all the records from the right DataFrame and the matching records from the left DataFrame.
- Example:
df1.join(df2, "commonKey", "right_outer")
- Full Outer Join:
- Full outer join returns all the records from both DataFrames and fills the missing values with null if there is no match.
- Example:
df1.join(df2, "commonKey", "outer")
- Left Semi Join:
- Left semi join returns only the records from the left DataFrame that have a match in the right DataFrame.
- Example:
df1.join(df2, "commonKey", "left_semi")
- Left Anti Join:
- Left anti join returns only the records from the left DataFrame that do not have a match in the right DataFrame.
- Example:
df1.join(df2, "commonKey", "left_anti")
- Cross Join (Cartesian Join):
- Cross join returns the Cartesian product of both DataFrames, resulting in every possible combination of rows.
- Example:
df1.crossJoin(df2)
4. How does Spark handle data shuffling? Explain the concept of shuffle operations.
Ans: Data shuffling is a crucial operation in distributed data processing, including Spark. It refers to the process of redistributing data across the cluster to facilitate operations that require data to be grouped, aggregated, or joined.
Let’s understand how Spark handles data shuffling and the concept of shuffle operations:
- Definition of Shuffle:
- Shuffle is a data reorganization process that occurs between stages in a Spark application.
- It involves the movement of data across the network, from multiple mapper nodes to reducer nodes, to perform tasks like grouping, aggregating, sorting, or joining.
- Shuffle Operations in Spark:
- Shuffle operations in Spark are triggered by transformations that require data to be redistributed, such as groupByKey(), reduceByKey(), sortByKey(), join(), and distinct().
- These transformations result in the reshuffling of data partitions across the cluster.
- Shuffle Process:
- When a shuffle operation is triggered, Spark breaks the data into partitions and distributes them across the worker nodes based on the defined key.
- The data is then shuffled and moved over the network, so that each key is co-located on the same node or set of nodes.
- The reducer nodes aggregate and combine the shuffled data partitions according to the specified operation.
- Shuffling Process Steps:
- Map Phase: Each mapper node processes a portion of the input data and assigns a key to each record.
- Partitioning: The records are partitioned based on the keys and sent to the appropriate reducer nodes.
- Sorting: The records within each partition are sorted by key, if required.
- Transfer: The shuffled data partitions are transferred over the network from mappers to reducers.
- Reduce Phase: The reducer nodes process the shuffled data partitions and perform the required operations.
- Shuffle Dependencies and Stages:
- Shuffle operations introduce dependencies between stages in a Spark application.
- A stage represents a set of tasks that can be executed in parallel.
- Shuffle dependencies determine the boundaries between stages, where the shuffle occurs, and data is exchanged between stages.
- Shuffle Performance Considerations:
- Shuffling involves substantial data movement across the network, which can be a performance-intensive operation.
- Spark provides mechanisms to optimize shuffle performance, such as tuning the shuffle buffer size, setting the number of reducers, using partitioning techniques, or enabling compression.
- Additionally, Spark’s pipelining optimization, achieved through lazy evaluation and pipelining of transformations, reduces the amount of data shuffled between stages.
5. What is the significance of SparkContext and SparkSession in Spark?
Ans:
- SparkContext:
- SparkContext (sc) is the entry point and the core component of Spark that establishes a connection with the cluster manager.
- SparkContext represents the connection to a Spark cluster and coordinates the execution of tasks on the cluster.
- It manages the distributed computing resources, such as CPU cores and memory, across the worker nodes in the cluster.
- SparkContext provides the functionality to create RDDs, broadcast variables, and accumulators.
- It is responsible for managing the execution environment, handling fault tolerance, and coordinating the execution of Spark tasks.
- SparkSession:
- SparkSession is an enhanced version of the earlier SparkContext and provides a unified entry point for interacting with Spark functionalities.
- SparkSession encapsulates SparkContext and provides a higher-level API for working with structured and unstructured data.
- It integrates Spark’s core features, SQL, DataFrame, Dataset APIs, and supports working with various data sources and executing SQL queries.
- SparkSession allows seamless integration with Hive, enabling access to Hive tables and executing Hive queries.
- It provides optimizations like Catalyst Query Optimizer for efficient query processing and Tungsten for improved memory management and execution speed.
- SparkSession simplifies the configuration and management of Spark application properties and resources.
- It supports the creation of DataFrame and Dataset objects, which provide higher-level abstractions for working with structured data.
6. How can you optimize Spark jobs for better performance?
Ans: Optimizing Spark jobs is crucial for achieving better performance and efficient resource utilization. Here are several strategies and techniques to optimize Spark jobs:
- Data Partitioning and Skew Handling:
- Partition data wisely to achieve better parallelism and load balancing across worker nodes.
- Use appropriate partitioning techniques, such as hash partitioning or range partitioning, based on the data distribution and query patterns.
- Handle data skew by identifying skewed keys and applying techniques like salting, bucketing, or using custom partitioners to distribute the skewed data evenly.
- Broadcast Variables:
- Use broadcast variables to efficiently share small read-only data across all worker nodes.
- Broadcast variables are cached on each worker node, reducing network communication and memory consumption.
- Data Serialization:
- Choose efficient serialization formats like Apache Avro, Apache Parquet, or Apache ORC to reduce storage space and improve I/O performance.
- Utilize DataFrame or Dataset APIs that provide built-in support for columnar storage formats.
- Caching and Persistence:
- Cache intermediate data or frequently accessed RDDs/DataFrames in memory using the
persist()
orcache()
methods. - Caching allows faster access to data, reducing disk I/O and recomputation.
- Cache intermediate data or frequently accessed RDDs/DataFrames in memory using the
- Monitoring and Profiling:
- Monitor Spark job performance using Spark’s built-in monitoring tools, such as the Spark UI or Spark application logs.
- Profile and identify performance bottlenecks using tools like Spark’s built-in profiling APIs, third-party profilers, or Spark’s performance monitoring libraries like Apache Sparklens.
7. Explain the concept of partitioning in Spark and its role in parallel processing.
Ans: Partitioning refers to the process of dividing data into smaller, manageable chunks called partitions, which can be processed independently by different tasks in parallel. Here’s how partitioning works and its role in parallel processing:
- Data Partitioning:
- Spark divides data into partitions to distribute them across the worker nodes in a cluster.
- Each partition is a logical unit of data that can be processed independently.
- Spark provides automatic partitioning for some data sources, like Hadoop Distributed File System (HDFS), or you can manually specify the partitioning scheme for custom data sources.
- Role in Parallel Processing:
- Partitioning enables parallel processing by allowing multiple partitions to be processed concurrently on different worker nodes.
- Each partition is processed by a separate task, and the tasks can execute in parallel across the cluster, utilizing the available CPU cores.
- Parallel processing improves the overall throughput and reduces the processing time for large-scale data processing tasks.
- Partitioning Strategies:
- Spark offers various partitioning strategies based on the nature of the data and the operations to be performed.
- Hash Partitioning: Data is partitioned based on a hash function applied to a specific column or key. It ensures that records with the same key are assigned to the same partition.
- Range Partitioning: Data is partitioned based on a specific range or criteria. It is often used for sorting or range-based queries.
- Custom Partitioning: Users can define their own partitioning logic by implementing the Partitioner interface in Spark.
- Data Skew and Load Balancing:
- Proper partitioning helps mitigate data skew issues and load balancing challenges.
- Data skew refers to an uneven distribution of data among partitions, which can lead to performance degradation.
- By choosing an appropriate partitioning strategy and handling data skew, you can ensure that the workload is evenly distributed across partitions and worker nodes.
- Operations and Optimization:
- Partitioning affects various operations in Spark, such as joins, aggregations, shuffles, and data locality.
- Well-partitioned data can improve join and aggregation performance by reducing data shuffling and network communication.
- Optimizing partitioning can enhance data locality, where data is processed on the same node where it is stored, reducing network overhead and improving performance.
8. How does Spark handle fault tolerance and data recovery?
Ans: Spark incorporates several mechanisms to handle fault tolerance and ensure data recovery in the event of failures. These mechanisms enable Spark to recover from failures gracefully and continue the execution of jobs without losing data or progress. Here’s how Spark handles fault tolerance and data recovery:
- Resilient Distributed Datasets (RDDs):
- RDDs are the fundamental data structure in Spark and play a crucial role in fault tolerance.
- RDDs are immutable and partitioned collections of data that can be recomputed in case of failures.
- Spark tracks the lineage of each RDD, which is the sequence of transformations that led to its creation.
- In the event of a failure, Spark can recompute the lost partitions of RDDs by using the lineage information and the original data source.
- Directed Acyclic Graph (DAG) and RDD Lineage:
- Spark constructs a directed acyclic graph (DAG) of the transformations applied to RDDs.
- The DAG represents the execution plan of the Spark job and contains the lineage information.
- If a worker node fails, Spark can use the lineage information to determine the lost partitions and recompute them from the available data.
- Data Persistence and Caching:
- Spark provides methods like
persist()
andcache()
to persist intermediate data or frequently accessed RDDs/DataFrames in memory. - By caching data, Spark reduces the need for recomputation in case of failures.
- Cached data can be efficiently recovered from memory, improving performance and fault tolerance.
- Spark provides methods like
- Cluster Manager Integration:
- Spark integrates with cluster managers like Apache Mesos, Hadoop YARN, or standalone mode to leverage their fault tolerance capabilities.
- Cluster managers monitor the health of worker nodes and can restart failed nodes or allocate new resources to recover from failures.
9. What are broadcast variables in Spark? How are they useful in distributed computing?
Ans: Broadcast variables in Spark are read-only variables that are cached and shared across all worker nodes in a cluster. They allow efficient and scalable distribution of large, read-only data structures to worker nodes, reducing network communication and improving the performance of distributed computing. Here’s how broadcast variables work and their usefulness in distributed computing:
- Broadcast Mechanism:
- When a variable is marked as a broadcast variable, Spark serializes it and distributes it to all worker nodes.
- The broadcast variable is cached in memory on each worker node, making it accessible to tasks running on that node.
- Usefulness in Distributed Computing:
- Efficient Data Sharing: Broadcast variables enable efficient sharing of large read-only data structures, such as lookup tables, configuration settings, or machine learning models, across all worker nodes.
- Reduced Network Traffic: By broadcasting the variable, Spark avoids sending the data over the network repeatedly for each task. Instead, each worker node retrieves the broadcast variable from its local cache, reducing network communication overhead.
- Example Use Cases:
- Lookup Tables: Broadcast variables are commonly used to distribute lookup tables or reference data that need to be accessed by multiple tasks, such as mapping tables or dictionaries.
- Machine Learning Models: In distributed machine learning, broadcast variables can be used to distribute trained models or feature transformations to worker nodes during prediction or scoring.
10. Explain the concept of accumulators in Spark and their purpose.
Ans: Accumulators are special variables in Spark that enable aggregating values across multiple tasks in a distributed environment.
They are used for tracking and aggregating information from worker nodes back to the driver program. Here’s how accumulators work and their purpose:
- Definition and Usage:
- An accumulator is a write-only variable that can only be incremented by the workers and read by the driver program.
- Accumulators are created on the driver program and then passed to worker nodes for updates during task execution.
- Worker nodes can only add values to the accumulator using the
+=
operation, and the driver program can read the accumulated value.
- Purpose of Accumulators:
- Tracking Global Metrics: Accumulators are primarily used to track and aggregate global metrics or statistics from worker nodes. Examples include counting the number of processed records, summing up values, or tracking the occurrence of specific events across the distributed tasks.
- Monitoring and Debugging: Accumulators provide a mechanism to collect and monitor important information or metrics during the execution of Spark jobs. They can be used for debugging, performance analysis, or understanding the behavior of distributed computations.
- Important Considerations:
- Accumulators are intended for implementing simple aggregations or counting tasks and should not be used for complex computations or non-associative operations.
- Spark guarantees the atomicity and fault tolerance of accumulators, ensuring that they behave correctly even in the presence of failures or retries.
- Accumulators are lazily evaluated, meaning their value is only updated when an action is triggered on the RDD or DataFrame. This allows Spark to optimize their execution.