Welcome to our new post Top Amazon Data Structure Interview Questions. here you will get the top 10 most-asked interview questions on data structure and algorithms in Amazon. Get interview-ready for Amazon with a tailored focus on data structure questions for candidates with around one year of experience. Elevate your preparation with a curated selection of top Amazon interview questions that delve into fundamental data structure concepts. Whether you’re tackling linked lists, binary trees, or hash tables, these questions will hone your problem-solving skills. Be equipped to discuss array vs. linked list distinctions, hash table operations, heap structures, and more. Strengthen your understanding of time and space complexities, dynamic programming, and recursion. This resource provides a strategic advantage for your Amazon interview readiness, ensuring you’re poised to excel in technical discussions. Master the essentials and confidently stand out as you approach your Amazon data structure interview.
It will help you to prepare the Top Amazon Data Structure Interview Questions.
To Read more Interview questions about JAVA, Python, Scala
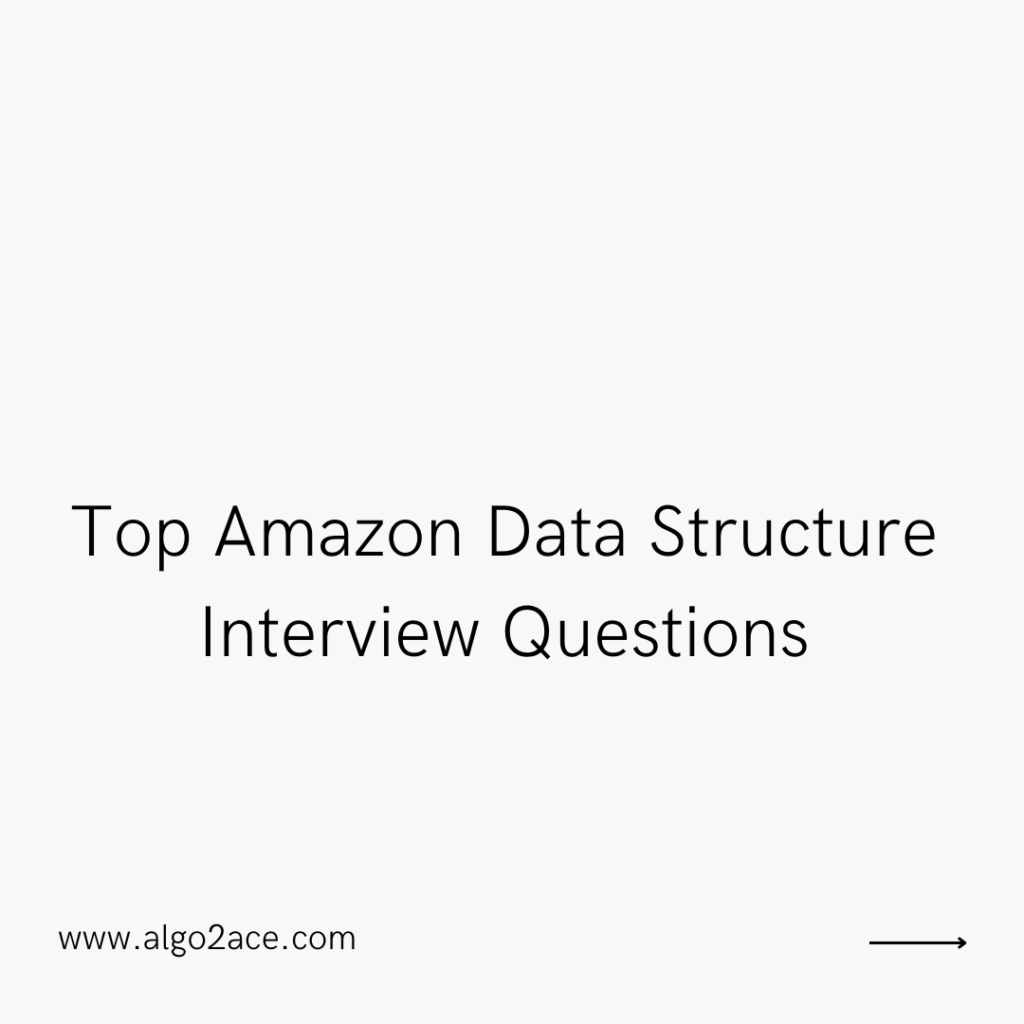
Top Amazon Data Structure Interview Questions
1. What is the difference between an array and a linked list? Explain the advantages and disadvantages of each data structure.
Aspect | Array | Linked List |
---|---|---|
Memory Allocation | Contiguous block of memory | Individual nodes with scattered memory |
Insertion/Deletion | Costly for inserts/deletes in the middle | Efficient for inserts/deletes anywhere |
Access Time | Constant time (O(1)) for random access | Linear time (O(n)) for sequential access |
Size Flexibility | Fixed-size; reallocation may be required | Dynamic size; can grow or shrink as needed |
Memory Overhead | Lower due to direct storage | Higher due to storing next pointers |
Cache Performance | Good due to memory locality | May suffer due to non-contiguous memory |
Usage | Best suited for frequent random access | Suitable for dynamic inserts/deletes |
Advantages of Arrays:
- Constant Time Access: Arrays provide direct access to elements using an index, resulting in continuous time access (O(1)).
- Memory Locality: Contiguous memory allocation leads to better cache performance, enhancing data retrieval speed.
- Simplicity: Arrays are simple to use and understand, making them a natural choice for straightforward scenarios.
Advantages of Linked Lists:
- Dynamic Size: Linked lists can grow or shrink dynamically, accommodating changing data requirements.
- Efficient Insertion/Deletion: Insertions and deletions at any point in the list are efficient, requiring only local changes.
- Memory Efficiency: Linked lists allow memory allocation for each node individually, minimizing memory wastage.
- No Preallocation Required: Linked lists don’t need preallocation, unlike arrays which often require resizing.
While arrays excel at random access and memory efficiency, linked lists shine when dealing with dynamic data and frequent insertions/deletions. The choice between them depends on the specific use case and performance requirements.
2. Explain the concept of time complexity and space complexity. How do you analyze the efficiency of an algorithm in terms of these complexities?
Time and space complexity are fundamental concepts used to analyze the efficiency of algorithms. They help us understand how an algorithm’s runtime and memory usage grow as the input size increases.
Time Complexity:
Time complexity measures the amount of time an algorithm takes to complete as a function of the input size. It’s typically expressed using Big O notation, which provides an upper bound on the growth rate of the algorithm’s runtime. For example, an algorithm with a time complexity of O(n) indicates that its runtime grows linearly with the size of the input.
To analyze time complexity:
- Identify the basic operations in the algorithm.
- Count the number of times each basic operation is executed as a function of the input size.
- Express the count in terms of Big O notation by dropping constants and lower-order terms.
Space Complexity:
Space complexity measures the amount of memory an algorithm uses as a function of the input size. It considers both the memory required for the algorithm’s instructions and the memory used by data structures, variables, and other auxiliary components.
To analyze space complexity:
- Identify the memory used by the algorithm’s variables, data structures, and other components.
- Sum up the memory used by each component as a function of the input size.
- Express the sum in terms of Big O notation.
Efficiency Analysis:
- Worst Case vs. Average Case: Algorithms may perform differently for different inputs. Analyze the worst-case scenario, which gives an upper bound on how the algorithm performs for any information.
- Dominant Terms: Focus on the most significant terms in the time and space complexity expressions. More minor terms and constants are dropped in Big O notation.
- Comparative Analysis: Compare the complexities of different algorithms solving the same problem to determine which one is more efficient in terms of time and space.
- Trade-offs: Sometimes, optimizing time complexity may lead to higher space complexity and vice versa. Analyze the trades based on the requirements of the problem.
- Asymptotic Analysis: Big O notation provides an asymptotic upper bound. It’s especially useful for analyzing how algorithms scale for large input sizes.
03. What is the time complexity of searching for an element in a sorted array using binary search?
The time complexity of searching for an element in a sorted array using binary search is O(log n), where “n” is the number of elements in the array.
Binary search works by repeatedly dividing the search interval in half until the desired element is found or it’s determined that the element is not present in the array. In each step of the algorithm, the search space is effectively halved. This logarithmic behavior means that the time it takes to complete the search increases very slowly as the size of the input (the array) grows. It’s significantly faster than linear search, which has a time complexity of O(n) for a sorted array.
04. Define a binary search tree (BST). How does it differ from a regular binary tree?
A Binary Search Tree (BST) is a specific type of binary tree data structure in which each node has at most two children, and the following properties hold:
a. Value Ordering: For each node in the BST:
- All nodes in its left subtree have values less than the node’s value.
- All nodes in its right subtree have values greater than the node’s value.
b. Unique Values:
All values stored in the BST are unique. No two nodes can have the same value.
A regular binary tree, on the other hand, does not have any specific ordering of values between nodes. In a regular binary tree, there are no restrictions on how values are organized within the tree, and there is no requirement for unique values among nodes.
To illustrate the difference, here’s an example:
Binary Search Tree (BST):
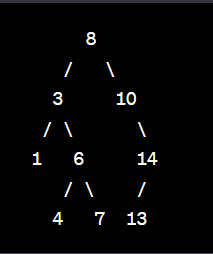
In this BST, the value ordering property holds. For any node, all values in its left subtree are less than the node’s value, and all values in its right subtree are greater.
Regular Binary Tree:
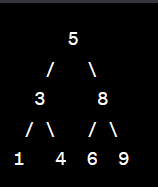
In this regular binary tree, there is no specific ordering of values between nodes. It’s not organized in a way that satisfies the BST property.
The key difference between a BST and a regular binary tree is that a BST is designed for efficient searching, insertion, and deletion of elements with a time complexity of O(log n) in average cases (assuming it’s reasonably balanced), while a regular binary tree does not have any inherent ordering, and its performance characteristics for these operations may not be as efficient.
05. What is the significance of a balanced binary tree, and why is it important in data structures?
A balanced binary tree, such as an AVL tree or a Red-Black tree, is a specific type of binary search tree (BST) that maintains a balance condition. In a balanced binary tree, the heights of the left and right subtrees of any node differ by at most one. This balance condition ensures that the tree remains relatively shallow and height-balanced.
The significance of a balanced binary tree and why it’s important in data structures can be understood through the following points:
a. Efficient Searching:
A balanced binary tree guarantees that the depth of the tree is logarithmic in the number of nodes. As a result, searching for an element in a balanced tree has an average and worst-case time complexity of O(log n), where “n” is the number of nodes. This is significantly faster than searching in an unbalanced binary tree, which can have a worst-case time complexity of O(n) in the worst case.
b. Efficient Insertion and Deletion:
Maintaining balance in a binary tree ensures that insertions and deletions can be performed efficiently in O(log n) time. This is crucial for data structures that require dynamic operations, such as sets, maps, and dictionaries.
c. Prevents Worst-Case Scenarios:
Without balance, a binary tree could degenerate into a linked list-like structure, where one subtree becomes much deeper than the other. In this worst-case scenario, searching, insertion, and deletion operations become inefficient, with a time complexity of O(n). Balanced trees prevent such worst-case scenarios.
06. Explain the concept of hashing. How is it used in data structures like hash tables?
Hashing is a technique used in computer science to map data of arbitrary size (such as keys or values) to fixed-size values, typically numerical values, known as hash codes or hash values. The primary goal of hashing is to efficiently store, retrieve, and manage data in various data structures, with a focus on achieving constant-time average-case complexity for key operations like insertion, deletion, and retrieval.
a. Hash Function:
- A hash function is a mathematical function that takes an input (or “key”) and returns a fixed-size hash code.
- The hash code is typically a numerical value, but it can be any fixed-size data (e.g., an integer or a bit string).
- The same input should always produce the same hash code (deterministic behavior).
b. Hashing in Data Structures:
- Hashing is commonly used in data structures, with one of the most popular applications being the hash table (also known as a hash map).
- A hash table is an array-based data structure that uses a hash function to map keys to array indices (buckets).
- Each bucket can store one or more key-value pairs.
c. Hashing Use Cases:
- Hashing is used in various data structures and algorithms, not just hash tables. For example, it’s used in hash-based sets and dictionaries, as well as in techniques like bloom filters, which provide fast membership tests.
- Hashing is also used in security applications, such as password storage (salting and hashing) and digital signatures.
07. What is dynamic programming, and in what type of problems is it typically applied?
Dynamic programming (DP) is a powerful algorithmic technique used in computer science and mathematics to solve problems by breaking them down into smaller overlapping subproblems and storing the solutions to these subproblems to avoid redundant computations. It’s particularly effective for optimization problems where you want to find the best solution among a set of possible solutions.
Dynamic programming is typically applied to problems falling into one of two categories:
a. Top-Down (Memoization):
In this approach, you start with the original problem and recursively break it down into smaller subproblems. You memorize (store) the solutions to these subproblems in a data structure (like a dictionary or an array) to avoid recomputing them when needed. This approach is known as memoization.
b. Bottom-Up (Tabulation):
In this approach, you start by solving the smallest subproblems first and use their solutions to build up to the original problem. You often use an array or a table to store solutions to subproblems, and you fill it in a systematic manner. This approach is known as tabulation.
08. What is the Big O notation, and how is it useful in analyzing algorithm efficiency?
The Big O notation is a mathematical notation used in computer science to describe the upper bound of an algorithm’s time complexity or space complexity in terms of the input size. It provides a way to analyze and compare the efficiency of algorithms while abstracting away constant factors and lower-order terms. Big O notation is useful for understanding how an algorithm’s performance scales as the input size grows.
Key points about Big O notation and its utility in analyzing algorithm efficiency:
a. Definition:
Big O notation, denoted as O(f(n)), represents an upper bound on the growth rate of a function in terms of the input size “n.” It describes how the algorithm’s resource usage (time or space) grows asymptotically as the input size increases.
b. Asymptotic Analysis:
Big O notation focuses on the behavior of an algorithm as the input size approaches infinity. It doesn’t concern itself with specific constants, lower-order terms, or input sizes that are not “large enough.” This simplification allows for a high-level understanding of efficiency trends.
c. Comparative Analysis:
Big O notation allows you to compare algorithms and make informed decisions about which one to choose for a particular problem. An algorithm with a lower-order Big O complexity is generally more efficient for large inputs.
d. Worst-Case Analysis:
Big O notation often describes the worst-case scenario for an algorithm. It provides an upper bound on how an algorithm behaves when dealing with the most unfavorable input.
09. Describe the differences between depth-first search (DFS) and breadth-first search (BFS) traversal algorithms in graphs.
Aspect | Depth-First Search (DFS) | Breadth-First Search (BFS) |
Traversal Order | LIFO (Last In, First Out) | FIFO (First In, First Out) |
Data Structure (Used for Queue) | Stack | Queue |
Nature of Traversal | Depth-first (explores as far as possible along each branch before backtracking) | Breadth-first (explores all neighbors at the current level before moving to the next level) |
Implementation Complexity | Typically implemented recursively or using an explicit stack. | Typically implemented using a queue data structure. |
Memory Usage | Can use less memory compared to BFS as it explores one branch completely before moving to the next. | Tends to use more memory, especially for wide graphs, as it stores all neighbors of a level before proceeding to the next level. |
Time Complexity | O(V + E) for an adjacency list representation (V: vertices, E: edges) in the worst case. | O(V + E) for an adjacency list representation in the worst case. |
10. What is memoization, and how can it improve the performance of recursive algorithms?
Memoization is an optimization technique used in computer programming to improve the performance of recursive algorithms, particularly those that involve repeated calculations of the same subproblems. It involves caching or storing the results of expensive function calls and reusing those results when the same inputs occur again, instead of recalculating them.
Here’s how memoization works and how it can improve the performance of recursive algorithms:
a. Caching Results:
When a recursive function is called with a particular set of input parameters, memoization involves checking whether the function has already computed and stored the result for those parameters. If it has, the cached result is returned immediately instead of re-computing it.
b. Storage Mechanism:
Memoization typically uses data structures like dictionaries, arrays, or hash tables to store computed results. The input parameters serve as keys, and the corresponding function results are stored as values.
c. Base Cases:
Recursive functions that use memoization still need to define base cases to terminate the recursion. Base cases are typically straightforward and return predefined results for simple inputs, preventing infinite recursion.
Reference: