Introduction:
Scala Interview Questions For 2 Years Of Experience
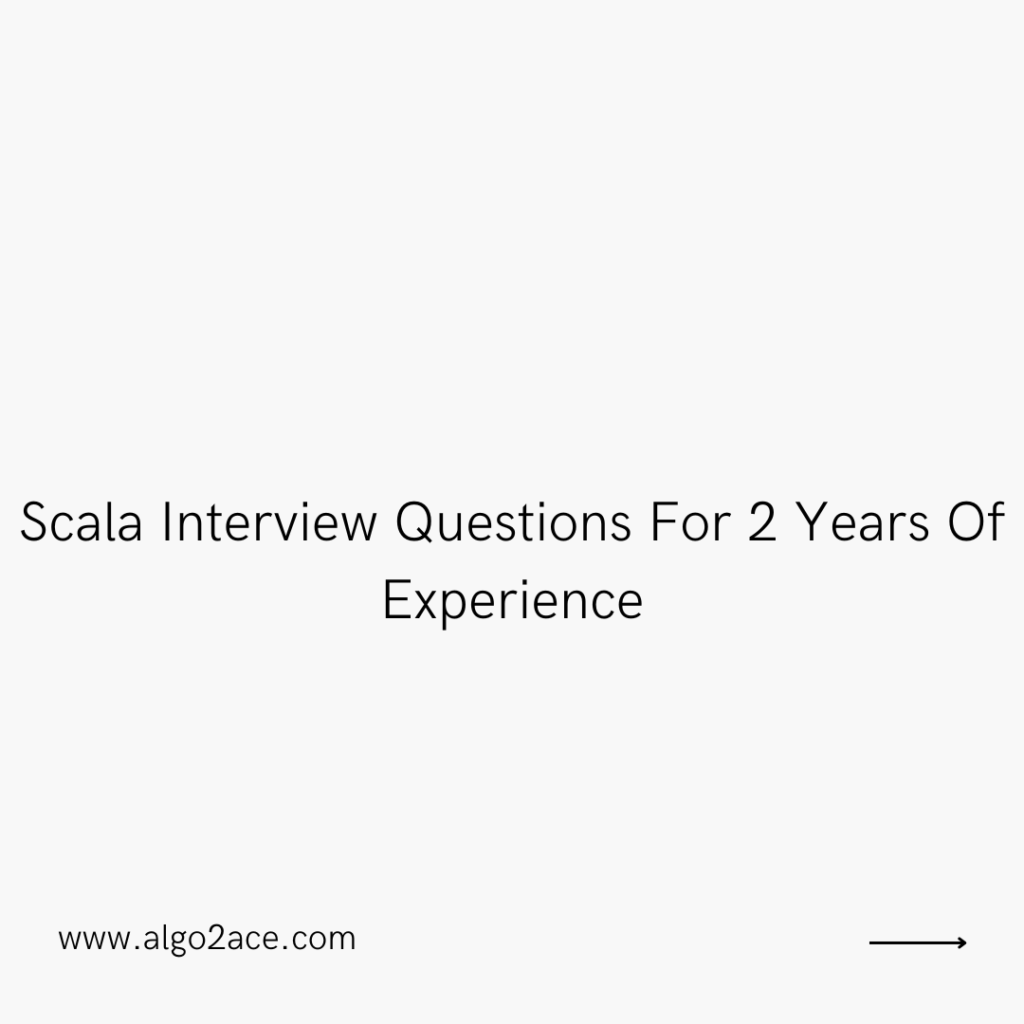
1. What is Scala? How does it differ from other programming languages?
Scala is a statically typed programming language that combines object-oriented and functional programming paradigms. It was designed to be a concise, expressive, and scalable language that runs on the Java Virtual Machine (JVM). Here are some key characteristics and differences of Scala compared to other programming languages:
Object-Oriented and Functional: Scala is both object-oriented and functional. It supports all the features of traditional object-oriented languages like classes, objects, inheritance, and encapsulation. Additionally, it provides powerful functional programming capabilities like higher-order functions, immutability, and pattern matching.
Static Typing: Scala is statically typed, which means that variable types are checked at compile time. It helps catch type-related errors early in the development process, leading to more robust and reliable code.
Conciseness: Scala emphasizes brevity and expressiveness. It has a concise syntax, allowing developers to write compact code that is readable and maintainable. It reduces boilerplate code and promotes a more productive development experience.
Interoperability: Scala runs on the JVM, which provides excellent interoperability with Java. Scala code can seamlessly call Java code and utilize existing Java libraries. This compatibility allows Scala developers to leverage the vast Java ecosystem.
Functional Programming Features: Scala incorporates functional programming concepts like immutability, higher-order functions, pattern matching, and support for immutable collections. These features enable developers to write concise and expressive code and take advantage of functional programming benefits like better concurrency support and code clarity.
2. Explain the concept of immutability in Scala and why it is important.
Immutability is a fundamental concept in functional programming, and Scala embraces it as a core principle. In Scala, immutability refers to the property of objects whose state cannot be changed after they are created.
There are several reasons why immutability is important in Scala and functional programming in general:
Simplified State Management: Immutability simplifies state management by eliminating the need for complex synchronization mechanisms and reducing the possibility of race conditions in concurrent code. Since immutable objects cannot be modified, multiple threads can safely access them without the risk of interference.
Thread Safety and Concurrency: Immutable data structures are inherently thread-safe, making it easier to write concurrent and parallel code. Immutable objects can be shared among multiple threads without the need for locks or synchronization, leading to more reliable and efficient concurrent programs.
Code Clarity and Debugging: Immutable code tends to be more readable and easier to reason about. Since the state of immutable objects cannot change, it becomes easier to understand their behavior and predict the outcome of operations performed on them. Debugging also becomes simpler because the state of objects remains constant, reducing the complexity of tracking down mutable state-related bugs.
3. What are higher-order functions in Scala? Provide an example.
In Scala, higher-order functions are functions that take other functions as parameters and/or return functions as results. They treat functions as first-class citizens, allowing them to be manipulated and passed around like any other value.
Here’s an example of a higher-order function in Scala:
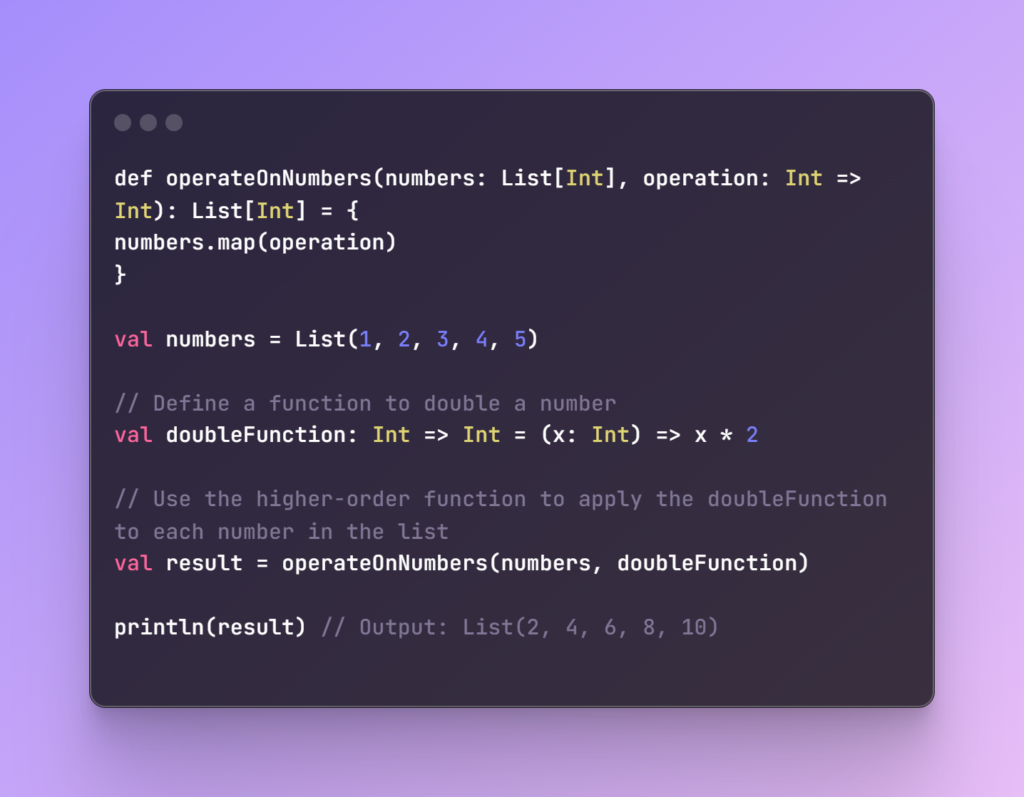
In this example, we have a higher-order function called operateOnNumbers. It takes two parameters: numbers, which is a list of integers, and operation, which is a function that takes an integer as input and returns an integer. Inside the operateOnNumbers function, we use the map function to apply the operation function to each element in the numbers list. The map function transforms each element of the list by applying the operation function to it.
4. Explain pattern matching in Scala and provide a use case for it.
Pattern matching is a powerful feature in Scala that allows you to match and extract data structures against patterns.
In Scala, pattern matching is performed using the match keyword followed by a series of case clauses. Each case clause specifies a pattern to match against and the corresponding code to execute if the pattern is matched. The patterns can include constants, variables, wildcards, constructors, guards, and other patterns.
Here’s a simple example to demonstrate pattern matching with an integer
In this example, we define a function matchNumber that takes an integer num as input. The function uses pattern matching to match the value of num against different cases. If num matches a particular case, the corresponding code block is executed. In this case, the input num is 2, so it matches the pattern 2, and the code block “Two” is executed, resulting in “Two” being returned.
Use Case: Pattern matching is commonly used in Scala for various scenarios, such as:
Deconstruction of case classes: Pattern matching allows you to destructure case classes and extract their values easily. This is especially useful when working with algebraic data types or modeling complex data structures.
Parsing and processing data: Pattern matching can be used to parse and process structured data, such as JSON or XML. Each pattern case can represent a different structure or data format, enabling you to extract and process the relevant parts of the data based on its shape.
Pattern matching with sealed traits: Sealed traits and pattern matching work well together to provide exhaustive handling of different types or states. Pattern matching can help ensure that all possible cases are covered, improving the reliability and maintainability of code.
5. What are traits in Scala? How are they different from abstract classes?
In Scala, traits are a fundamental language construct used to define reusable units of behavior that can be mixed into classes.
Traits in Scala:
Traits in Scala are similar to interfaces in other languages but with the ability to provide method implementations.
A trait is defined using the trait keyword, followed by the trait name and its body, which contains method declarations, field declarations, and default implementations.
Traits can be mixed into classes using the extends or with keyword. A class can inherit multiple traits, enabling multiple inheritance of behavior.
Differences from Abstract Classes:
Abstract classes in Scala can have constructors, whereas traits cannot have constructors.
A class can extend only one abstract class but can mix in multiple traits. This allows for multiple inheritance of behavior through traits.
Abstract classes are designed for inheritance-based hierarchies, while traits are designed for mixin-based composition.
6. How does Scala handle exceptions? Explain the try-catch-finally block.
In Scala, exceptions are handled using the try-catch-finally block, which is similar to exception handling in many other programming languages.
Here’s how the try-catch-finally block works in Scala:
try: The try block contains the code that may throw an exception. It is the portion of code where you want to handle potential exceptions. If an exception occurs within the try block, the control flow immediately jumps to the corresponding catch block.
catch: The catch block is used to catch and handle specific exceptions. It follows the try block and contains code that executes when an exception is thrown. Scala provides pattern matching within catch blocks, allowing you to match different exception types and handle them differently.
You can have multiple catch blocks following the try block, each handling a specific exception type. The first catch block that matches the exception type will be executed, and subsequent catch blocks will be skipped.
You can also use a general catch block without specifying any exception type to handle any unexpected exception.
finally: The finally block is optional and is used to specify code that should always be executed, regardless of whether an exception occurred or not. It is typically used for cleanup operations like closing resources or releasing acquired locks. The code in the finally block executes after the try and catch blocks have completed.
Here’s an example that demonstrates the usage of the try-catch-finally block in Scala:
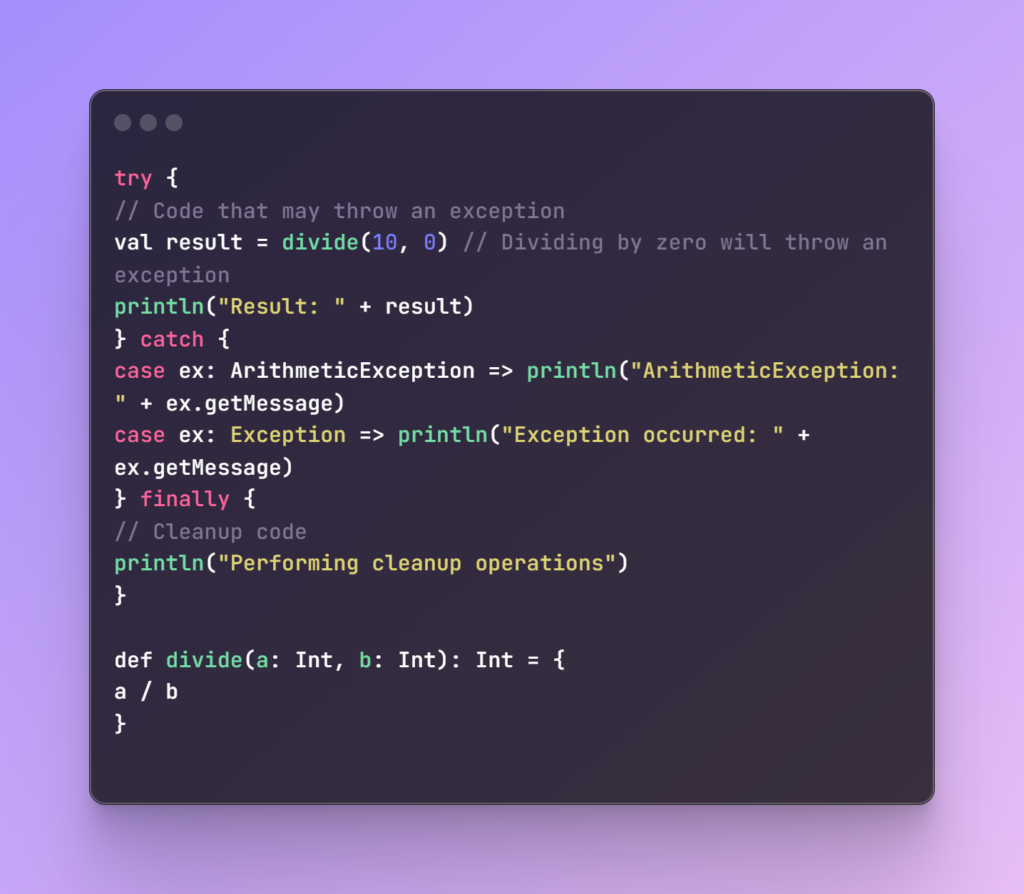
7. What is currying in Scala? Provide an example.
Currying is a technique in functional programming where a function that takes multiple arguments is transformed into a sequence of functions, each taking a single argument.
In currying, a function that takes multiple arguments is transformed into a chain of functions, where each function takes a single argument and returns a new function that takes the next argument. This process continues until all the arguments are consumed, and the final function returns the result.
Here’s an example to demonstrate currying in Scala:
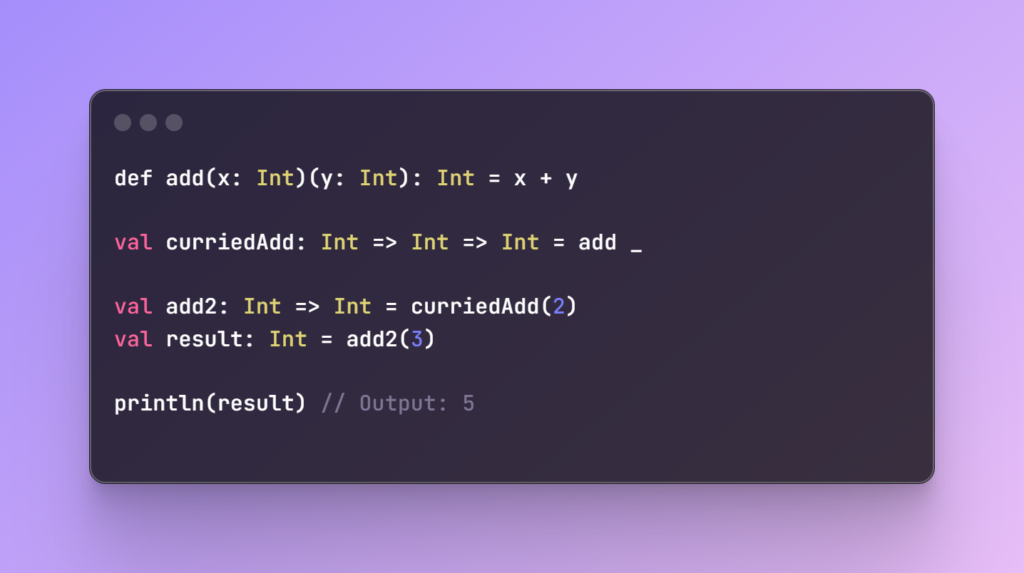
In the above example, the add function is defined with currying. It takes two arguments, x and y, but it is split into two argument lists. The first argument list is (x: Int), and the second argument list is (y: Int). The function body simply adds the two arguments.
By using curriedAdd(2), we create a specialized version of the add function where the first argument x is fixed to 2. This results in a new function add2 that takes a single argument y and returns the sum of 2 and y.
Finally, we call add2(3), which passes 3 as the argument to the add2 function, and the result is computed as 2 + 3, yielding 5.
8. How does implicit conversion work in Scala?
Implicit conversions in Scala allow the compiler to automatically convert one type to another type when required.
Implicit conversions are defined using implicit functions or implicit classes. An implicit function is a regular function that is marked with the implicit keyword, and an implicit class is a special kind of class that is also marked with the implicit keyword.
Here’s a simple example to illustrate how implicit conversion works:
case class Celsius(value: Double)
case class Fahrenheit(value: Double)
object TemperatureConverter {
implicit def celsiusToFahrenheit(celsius: Celsius): Fahrenheit =
Fahrenheit(celsius.value * 9 / 5 + 32)
}
import TemperatureConverter._
val temperatureCelsius: Celsius = Celsius(30.0)
val temperatureFahrenheit: Fahrenheit = temperatureCelsius
println(temperatureFahrenheit) // Output: Fahrenheit(86.0)
9. Explain the concept of futures and promises in Scala for asynchronous programming.
Futures: A future represents a result that may not be available yet. It is a placeholder for a value that will be computed asynchronously. The result of a future can be obtained once it is available, enabling non-blocking operations.
To create a future, you can use the Future object’s apply method or various combinators. The future represents the computation that will be executed concurrently in a separate thread or thread pool. You can attach callbacks to a future to be executed when the future completes successfully or fails.
Here’s an example of using a future to perform an asynchronous computation:
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
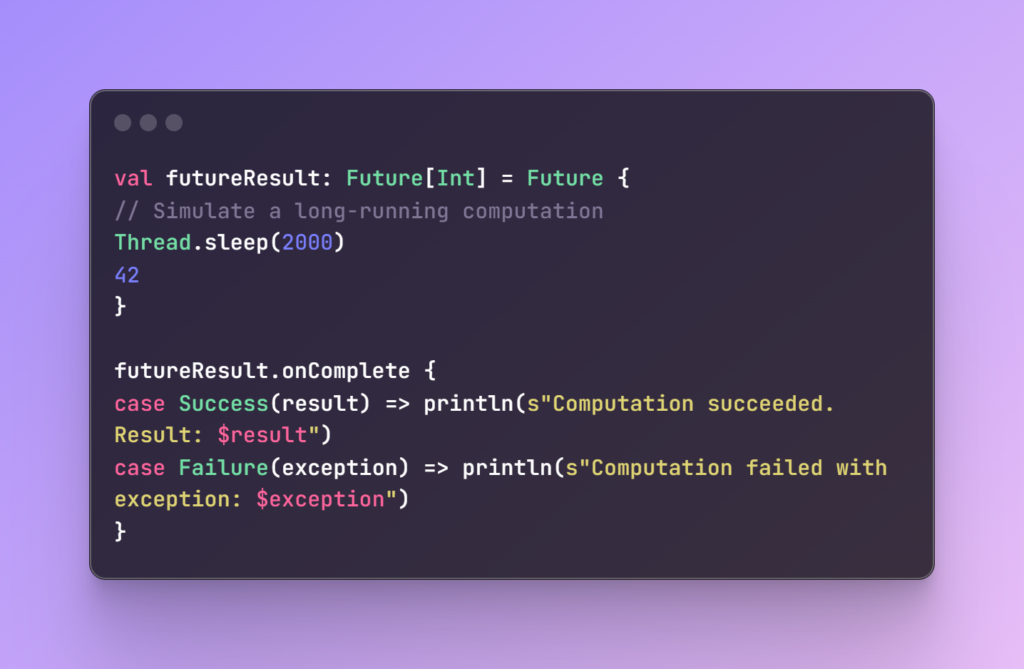
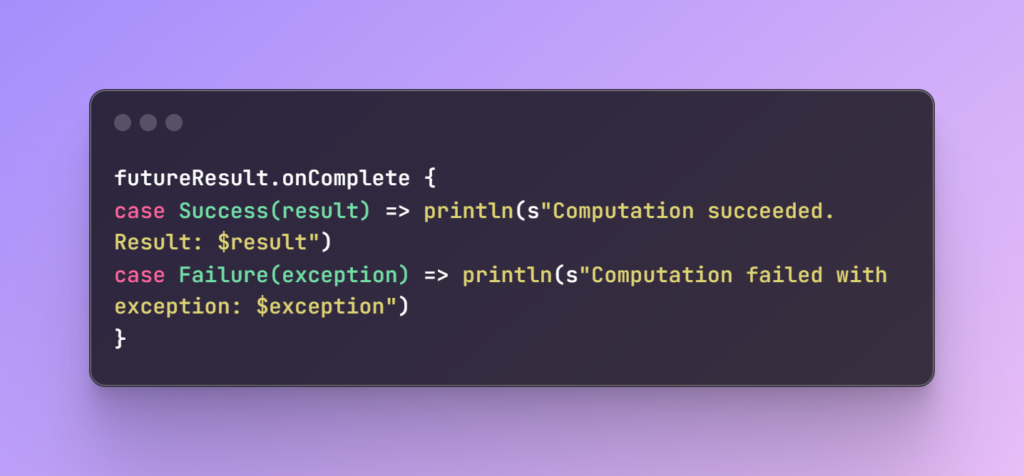
Promises: A promise is a writable, single-assignment container for a value that will be produced in the future. It is a way to create and control the completion of a future manually.
To create a promise, you can use the Promise object’s apply method. The promise provides methods to complete it successfully with a value (success), complete it with a failure (failure), or complete it with another future (completeWith).
Here’s an example of using a promise to complete a future:
import scala.concurrent.{Future, Promise}
import scala.concurrent.ExecutionContext.Implicits.global
val promiseResult: Promise[Int] = Promise[Int]()
val futureResult: Future[Int] = promiseResult.future
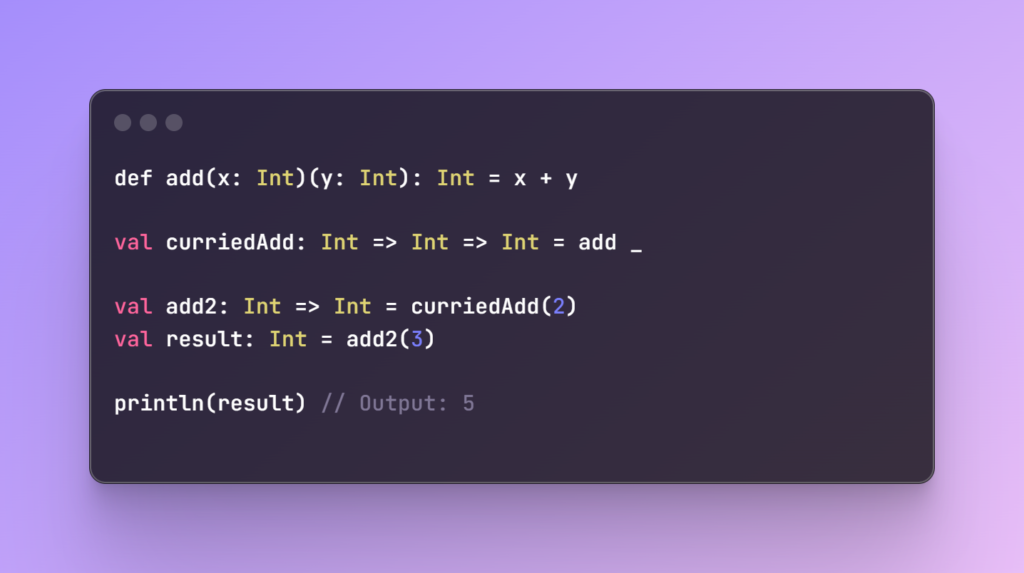
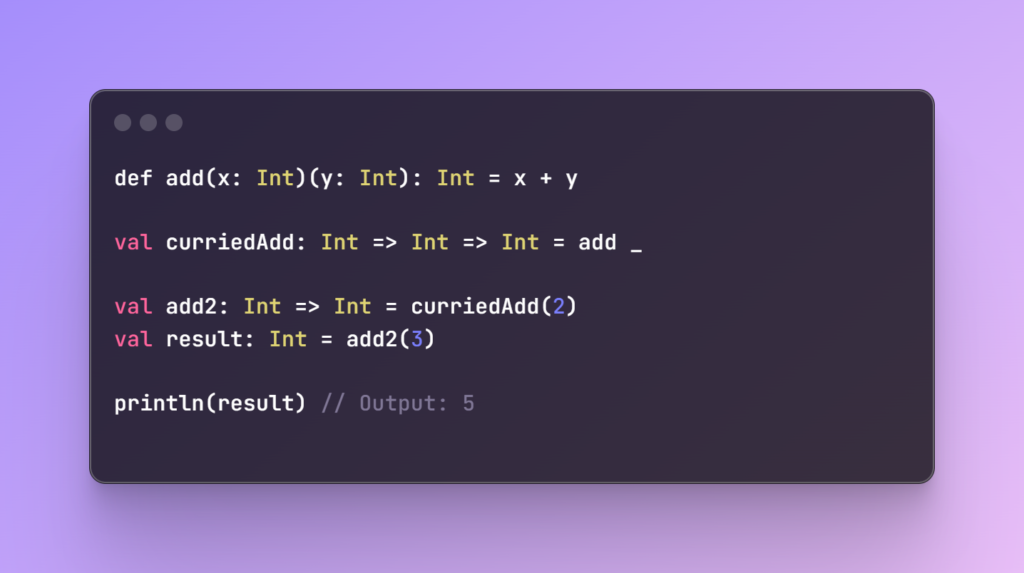
Here’s an example of using a promise to complete a future:
import scala.concurrent.{Future, Promise}
import scala.concurrent.ExecutionContext.Implicits.global
val promiseResult: Promise[Int] = Promise[Int]()
val futureResult: Future[Int] = promiseResult.future
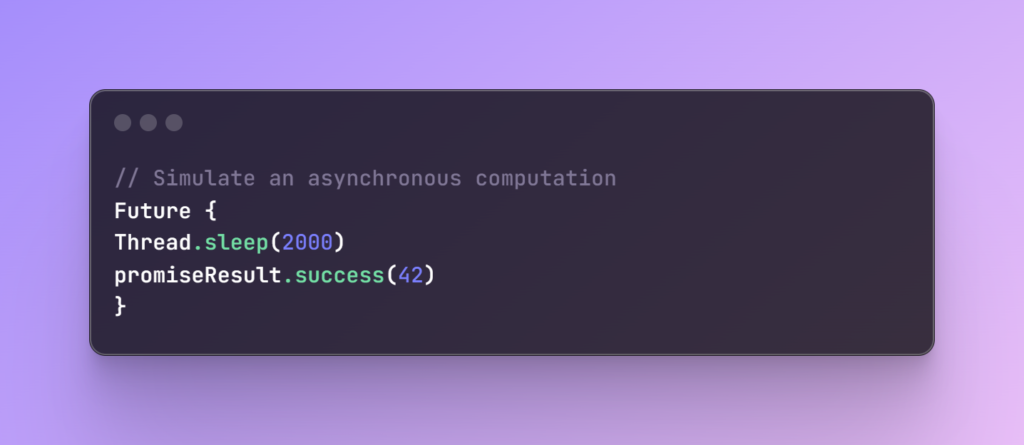
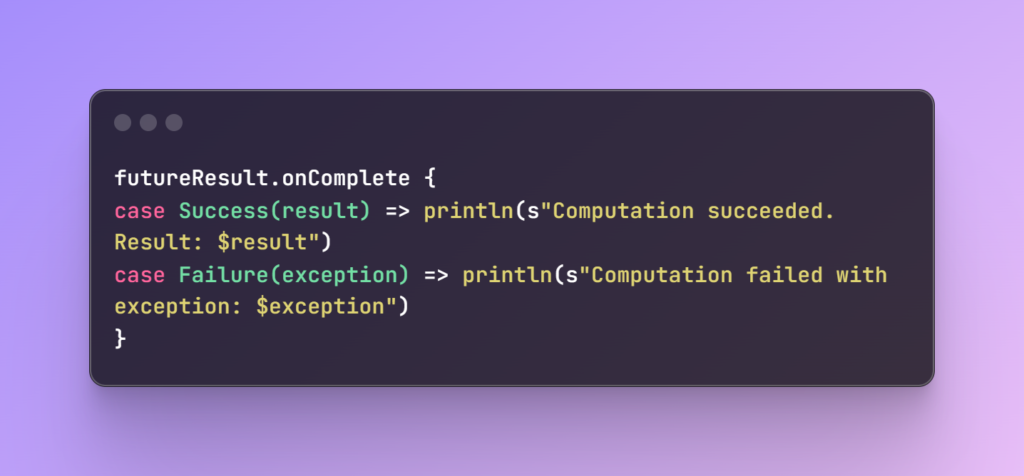
10. What is lazy evaluation in Scala? Provide a scenario where it can be beneficial.
Lazy evaluation is a feature in Scala that delays the evaluation of an expression until it is actually needed.
In Scala, lazy evaluation is achieved using the lazy keyword. By marking a variable or expression as lazy, its evaluation is postponed until it is accessed for the first time. The lazy value is computed only once, and subsequent accesses retrieve the cached value without re-evaluating the expression.
Here’s an example to illustrate lazy evaluation:
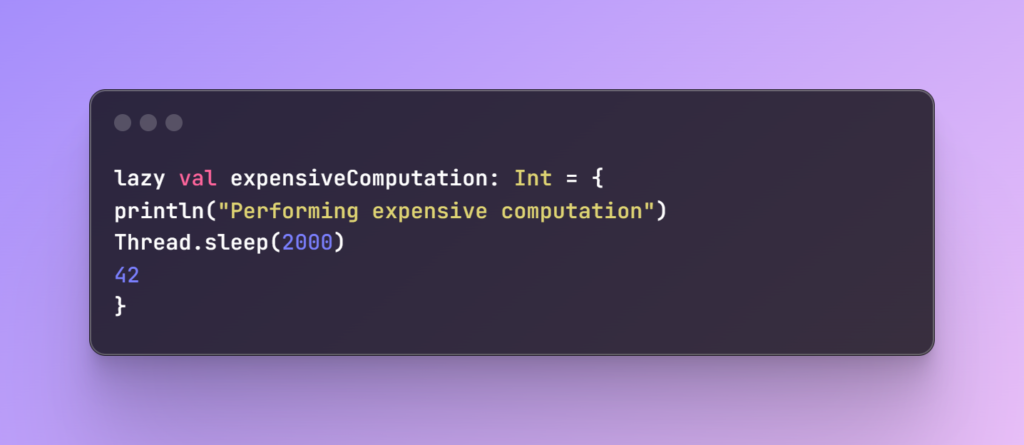
println(“Before accessing lazy value”)
println(expensiveComputation) // Expensive computation is performed here
println(“After accessing lazy value”)
println(expensiveComputation) // Cached value is retrieved without re-computation’
In this example, we define a lazy val named expensiveComputation. The expression assigned to expensiveComputation performs a time-consuming computation, simulated with Thread.sleep, and returns the value 42.
When we run the code, we notice that the “Performing expensive computation” message is printed only when we access expensiveComputation for the first time. The lazy evaluation defers the computation until it is actually needed. Subsequent accesses to expensiveComputation retrieve the cached value without re-performing the computation.