Introduction:
Welcome to the new post-JavaScript Interview Questions For 2 Years Experience.
In the dynamic landscape of web development, possessing a solid foundation in JavaScript is more critical than ever. For professionals with around two years of experience, navigating the intricacies of this versatile language becomes pivotal in ensuring success in job interviews.
This blog aims to empower developers by presenting 15 JavaScript interview questions tailored for individuals with approximately two years of hands-on experience. Each question is designed to assess a candidate’s understanding of essential concepts and their ability to apply JavaScript in real-world scenarios.
Whether you’re preparing for an upcoming interview or looking to strengthen your expertise, these questions serve as a valuable resource to bolster your proficiency in JavaScript.
JavaScript Interview Questions For 2 Years Experience
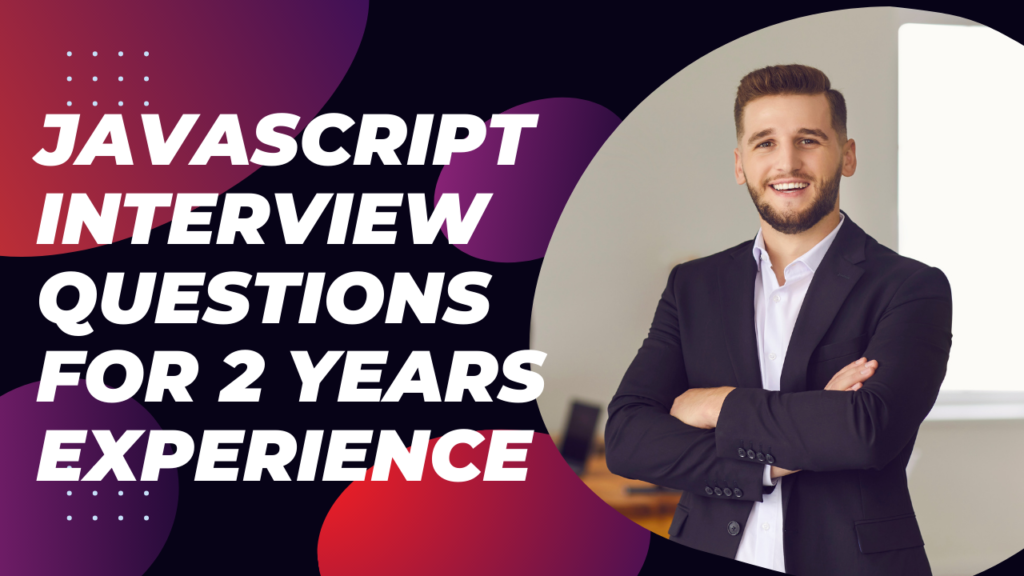
Certainly! Here are 15 JavaScript interview questions suitable for candidates with around 2 years of experience, along with their answers:
1. Explain the difference between `let`, `const`, and `var` in JavaScript.
`let` and `const` are block-scoped variables introduced in ES6, while `var` is function-scoped. `let` allows reassignment, `const` is used for constants, and `var` can be reassigned.
2. What is the significance of closures in JavaScript? Can you provide an example?
Closures allow functions to retain access to variables from their lexical scope, even after the function has finished executing. Example:
javascript
function outer() {
let outerVariable = 10;
function inner() {
console.log(outerVariable);
}
return inner;
}
let closureFunction = outer();
closureFunction(); // Outputs 10
3. How does asynchronous programming work in JavaScript, and what are some common patterns for handling it?
Asynchronous programming is handled using callbacks, promises, and async/await. Callbacks are functions passed as arguments, promises represent the eventual result of an asynchronous operation, and async/await provides a cleaner syntax for working with promises.
4. Explain the concept of event delegation.
Event delegation is a technique where a single event listener is attached to a common ancestor rather than individual elements. It takes advantage of event bubbling to handle events on child elements efficiently.
5. What is the difference between `==` and `===` in JavaScript?
`==` performs type coercion, converting operands to the same type before making the comparison. `===` (strict equality) does not perform type coercion and checks both value and type equality.
6. How can you handle errors in JavaScript?
Errors can be handled using try-catch blocks. The `try` block contains the code that might throw an exception, and the `catch` block handles the exception.
7. Explain the concept of hoisting.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase.
8. What is the purpose of the `this` keyword in JavaScript, and how is it determined in different contexts?
`this` refers to the current execution context. Its value is determined by how a function is called. In a method, it refers to the object on which the method is invoked.
9. What are arrow functions in JavaScript, and how do they differ from regular functions?
Arrow functions have a more concise syntax and do not have their own `this` binding. They inherit `this` from the enclosing scope.
10. Explain the concept of destructuring in JavaScript.
Destructuring allows you to extract values from arrays or objects and assign them to variables in a concise manner.
11. What is the purpose of the `map()` function in JavaScript?
The `map()` function creates a new array by applying a provided function to each element of the array. It does not modify the original array.
12. How can you achieve object-oriented programming (OOP) concepts in JavaScript?
JavaScript supports OOP concepts through prototypes. Objects can inherit properties and methods from other objects through their prototype chain.
13. Discuss the role of promises in handling asynchronous operations.
Promises represent the eventual completion or failure of an asynchronous operation. They simplify the handling of asynchronous code, providing a cleaner and more readable syntax.
14. Explain the concept of the event loop in JavaScript.
The event loop is a mechanism that allows JavaScript to perform non-blocking I/O operations by handling events and executing callback functions. It consists of a call stack, callback queue, and event loop.
15. What is the purpose of the `localStorage` and `sessionStorage` objects?
Both `localStorage` and `sessionStorage` are web storage objects that allow developers to store key/value pairs in a web browser. `localStorage` persists even after the browser is closed, while `sessionStorage` is limited to a session.
Reference:
Javascript Scenario-Based Interview Questions[Answered]
Conclusion:
Mastering JavaScript is an ongoing journey marked by continuous learning and adaptability. As you delve into the world of interview preparation, these 15 questions provide a holistic examination of your JavaScript prowess. From fundamental concepts like variable declaration and asynchronous programming to more advanced topics such as closures and the event loop, this set of questions is crafted to evaluate your practical knowledge.
As you navigate through these inquiries, remember that each answer is an opportunity to showcase not just what you know but how effectively you can apply that knowledge.
Whether you’re seeking new opportunities or aiming to enhance your current role, these JavaScript interview questions will undoubtedly contribute to your success in the ever-evolving field of web development. Embrace the challenge, stay curious, and let your journey with JavaScript continue to flourish.