Introduction:
JavaScript Interview Questions And Answers
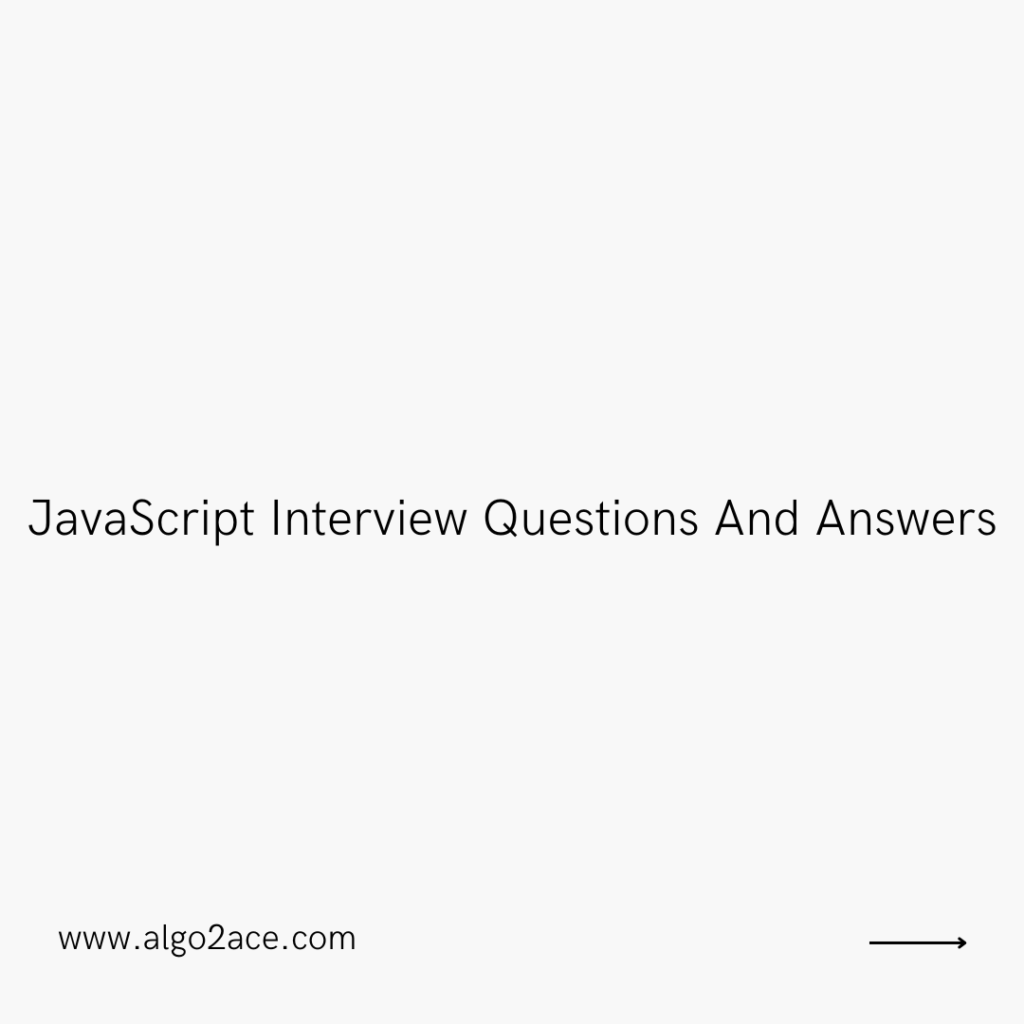
1. What is JavaScript?
JavaScript is a high-level, interpreted programming language that is primarily used for front-end web development. It was created to add interactivity and dynamic behavior to web pages. JavaScript is a versatile language that can be used for both client-side and server-side development.
2. What is the difference between null and undefined in JavaScript?
null:
null is a value that represents the intentional absence of any object value. It is a primitive value.
It is often used to indicate that a variable or object property has no value or is intentionally set to no value.
When a variable is assigned null, it means it has been explicitly assigned to represent nothing.
null is of type ‘object’.
When comparing to other values using the strict equality operator (===), null only equals undefined, and no other value.
Example: let myVariable = null;
undefined:
undefined is a value that indicates an uninitialized variable, a missing function argument, or an absent object property.
It is a global property that represents the default value of uninitialized variables and function parameters.
When a variable is declared but not assigned a value, it is automatically assigned the value undefined.
undefined is of type ‘undefined’.
When comparing to other values using the strict equality operator (===), undefined only equals null, and no other value.
Example: let myVariable;
3. Explain the concept of hoisting in JavaScript.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their respective scopes during the compilation phase, before the actual execution of the code.
Variable Hoisting:
When variables are hoisted, the variable declarations are moved to the top of their scope, but not the assignments.
This allows you to use variables before they are declared in the code, although it is considered a best practice to declare variables before using them.
If a variable is declared multiple times within the same scope, only the first declaration is hoisted. Subsequent declarations do not override the previous ones.
Example: console.log(myVariable); // Output: undefined var myVariable = 10;
Function Hoisting:
Function declarations are also hoisted to the top of their scope, allowing you to call functions before they are defined in the code.
Unlike variables, the entire function declaration is hoisted, including the function body.
This means you can call a function even before its actual declaration in the code.

4. What are closures in JavaScript and how do they work?
In JavaScript, a closure is a combination of a function and the lexical environment within which that function was declared. It allows a function to access variables from an outer function scope even after the outer function has finished executing. In other words, a closure retains access to its own scope, as well as the scope of its parent function and any other outer scopes.
Closures are created in JavaScript when an inner function is defined inside an outer function and then returned or accessed outside of that outer function. The inner function maintains a reference to its outer function’s scope, even if the outer function has completed execution.
Here’s an example to illustrate closures in JavaScript:
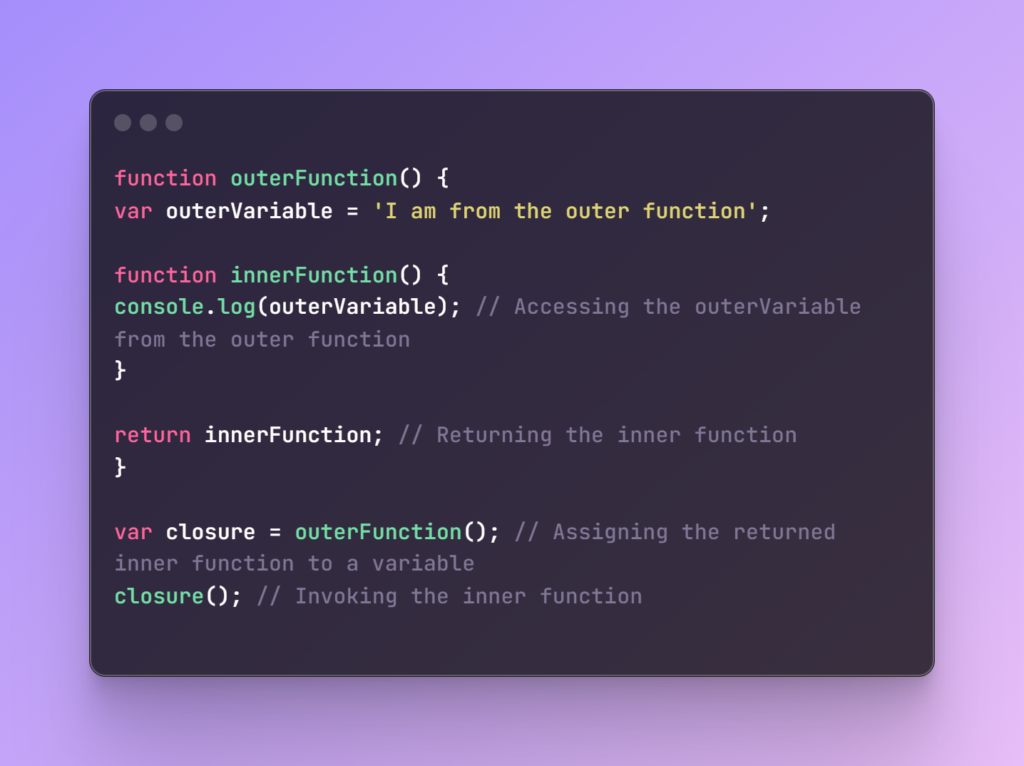
In the above example, the outerFunction defines an inner function called innerFunction that references the outerVariable from its parent scope. When outerFunction is called and the inner function is returned, the returned function still has access to the outerVariable due to closure. When closure() is invoked, it logs the value of outerVariable from the outer function, even though the outer function has already finished executing.
5. What is the difference between let, const, and var for variable declaration?
Scope Hoisting Redeclaration Reassignment
var Function scope Hoisted Allowed Allowed
let Block scope Not hoisted Not allowed Allowed
const Block scope Not hoisted Not allowed Not allowed
Please note that while let and const have similar behavior regarding hoisting and block scope, the key difference lies in their reassignment and redeclaration rules. let allows reassignment within the same scope but disallows redeclaration, whereas const disallows both reassignment and redeclaration once the variable is defined.
6. Explain the event delegation in JavaScript.
Event delegation is a technique in JavaScript where instead of attaching an event listener to each individual element, you attach a single event listener to a parent element that will handle events for all its descendants. When an event occurs on a descendant element, the event bubbles up through the DOM hierarchy and triggers the event listener attached to the parent element.
The concept of event delegation relies on event propagation, specifically the capturing and bubbling phases of the event flow. During the bubbling phase, the event moves from the target element to its parent elements up to the document level. By leveraging this bubbling behavior, you can listen for events on a parent element and handle them based on the target element that triggered the event.
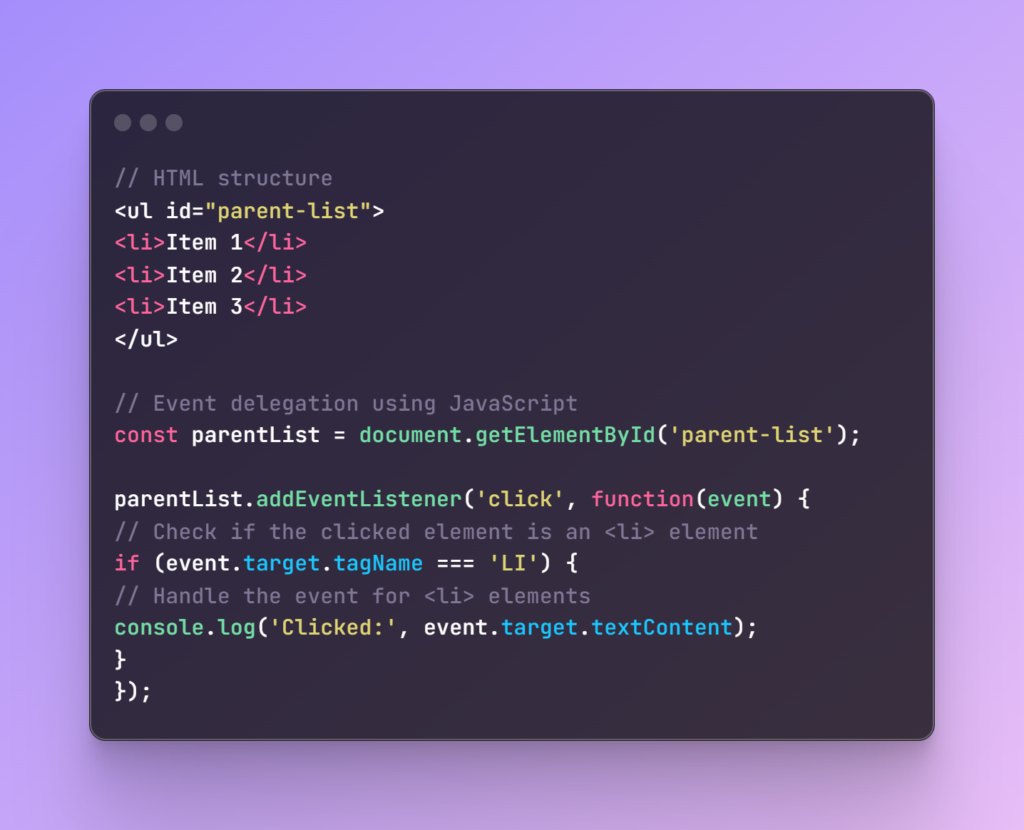
7. What is the difference between synchronous and asynchronous programming in JavaScript?
Aspect Synchronous Programming Asynchronous Programming
Execution Flow Sequential and blocking execution Non-blocking execution
Blocking Behavior Blocks execution until a task completes Does not block execution, allows concurrent tasks
Program Responsiveness Program may become unresponsive during long-running tasks Program remains responsive, allows concurrent operations
Task Completion Order Tasks are executed in a predictable order Tasks may complete in different order
Error Handling Immediate error handling through try-catch blocks Error handling through callbacks, promises, or async/await
Callbacks/Promises Relies on callbacks Supports both callbacks and promises
Code Readability May lead to callback hell and nested functions Promotes cleaner code with promises and async/await
Performance May suffer from performance issues due to blocking operations Can improve performance with non-blocking operations
Use Cases Simple and synchronous operations, small-scale applications Asynchronous tasks, time-consuming operations
8. How does prototypal inheritance work in JavaScript?
In JavaScript, prototypal inheritance is a mechanism by which objects can inherit properties and methods from other objects. It is based on the concept of prototypes, where objects can serve as templates for creating new objects.
Here’s how prototypal inheritance works in JavaScript:
Every object in JavaScript has an internal property called [[Prototype]] (also known as the “dunder proto” property).
The [[Prototype]] property references another object, which is called the prototype of the object.
When accessing a property or method on an object, JavaScript first checks if the object itself has that property. If not, it looks up the prototype chain.
The prototype chain is formed by linking objects through their [[Prototype]] property. It creates a hierarchical structure where objects inherit properties and methods from their prototypes.
If the property or method is found in one of the prototypes in the chain, it is accessed and used.
If the property or method is not found in any prototype in the chain, JavaScript returns undefined.
Here’s an example to illustrate prototypal inheritance:
// Parent object constructor
function Animal(name) {
this.name = name;
}
// Method defined on the prototype of the Animal constructor
Animal.prototype.sayHello = function() {
console.log(“Hello, I’m ” + this.name);
};
// Child object constructor
function Dog(name, breed) {
Animal.call(this, name); // Call the parent constructor to initialize inherited properties
this.breed = breed;
}
// Inherit properties and methods from Animal
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
// Method defined on the prototype of the Dog constructor
Dog.prototype.bark = function() {
console.log(“Woof!”);
};
// Create a new Dog object
var myDog = new Dog(“Buddy”, “Labrador”);
myDog.sayHello(); // Output: Hello, I’m Buddy
myDog.bark(); // Output: Woof!
In the above example, the Animal constructor function acts as the parent object, and the Dog constructor function acts as the child object inheriting from Animal. By linking the prototypes using Object.create(), the Dog objects inherit the sayHello() method from the Animal prototype.
9. What is the purpose of the this keyword in JavaScript?
In JavaScript, the this keyword refers to the current execution context, which is determined by how a function is called. It is a special variable that holds a reference to an object, and its value can change dynamically depending on the context of the function invocation.
10. What are JavaScript promises and how do they work?
JavaScript Promises are objects used for asynchronous programming. They represent the eventual completion or failure of an asynchronous operation and allow you to handle the result or error once it becomes available.
Promises have three states:
Pending: Initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully, and the promise is resolved with a value.
Rejected: The operation encountered an error, and the promise is rejected with a reason or error message.
Here’s an example that demonstrates the basic usage of Promises:
11. How do you handle errors and exceptions in JavaScript?
In JavaScript, you can handle errors and exceptions using try-catch blocks. The try-catch block allows you to catch and handle exceptions that may occur during the execution of your code.
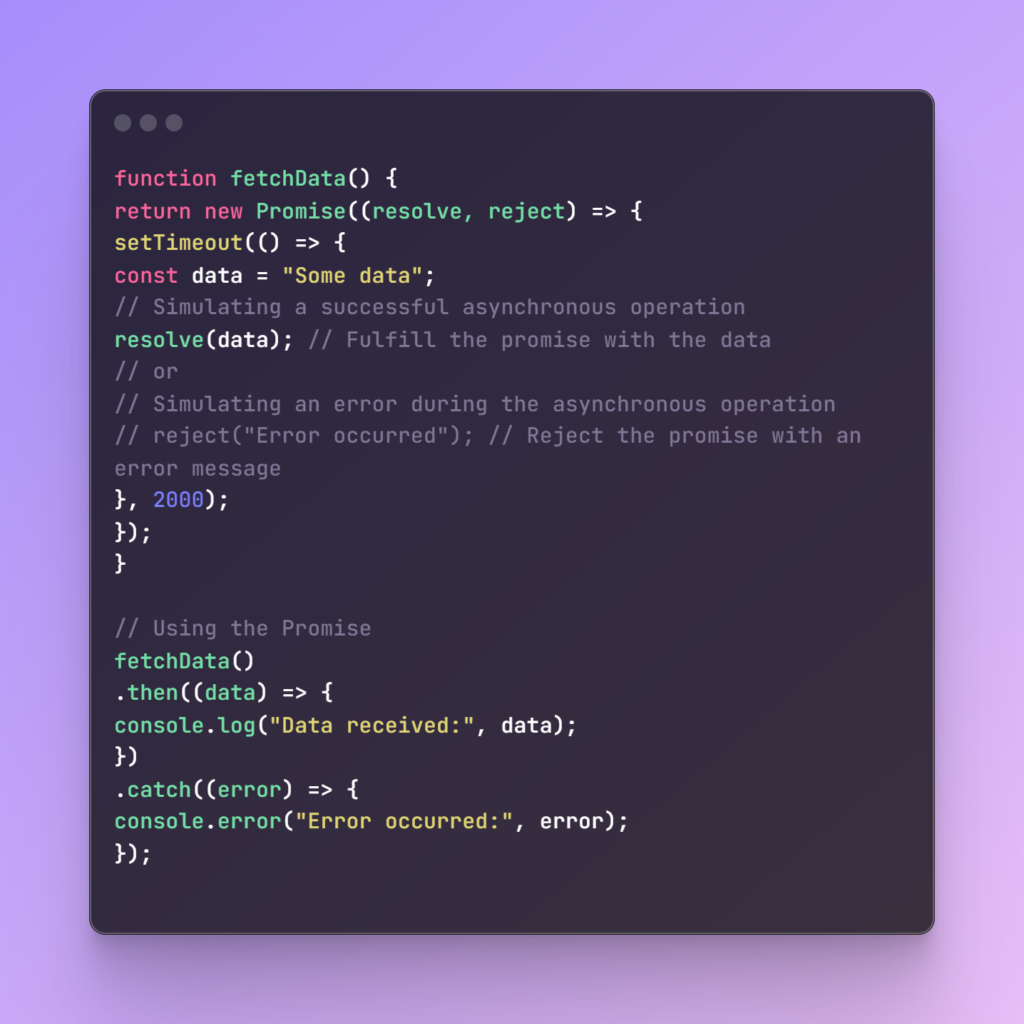
Here’s the basic syntax of a try-catch block:
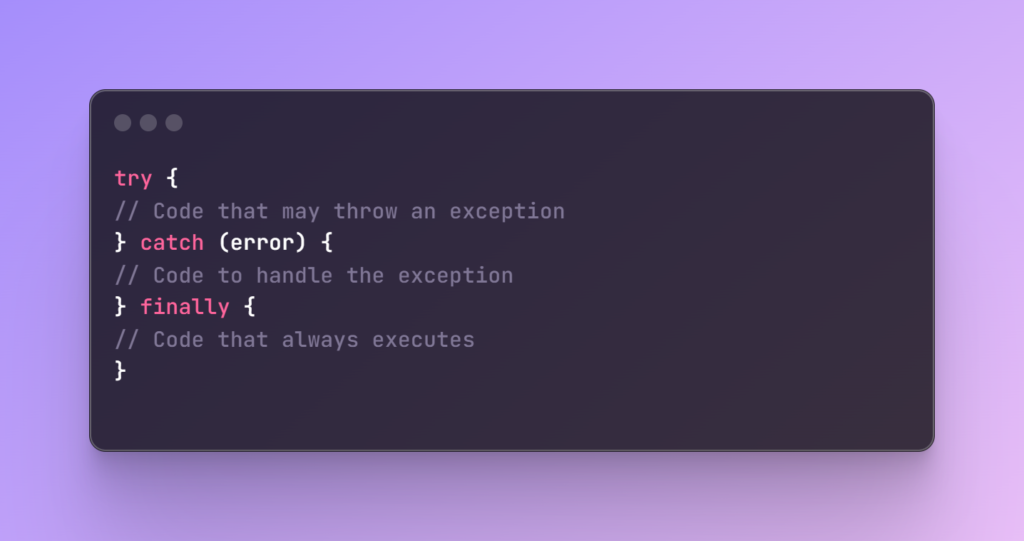
Within the try block, you write the code that you suspect may throw an exception. If an exception occurs within the try block, the catch block is executed. The catch block takes an error parameter, which represents the exception object.
Here’s an example that demonstrates error handling in JavaScript:
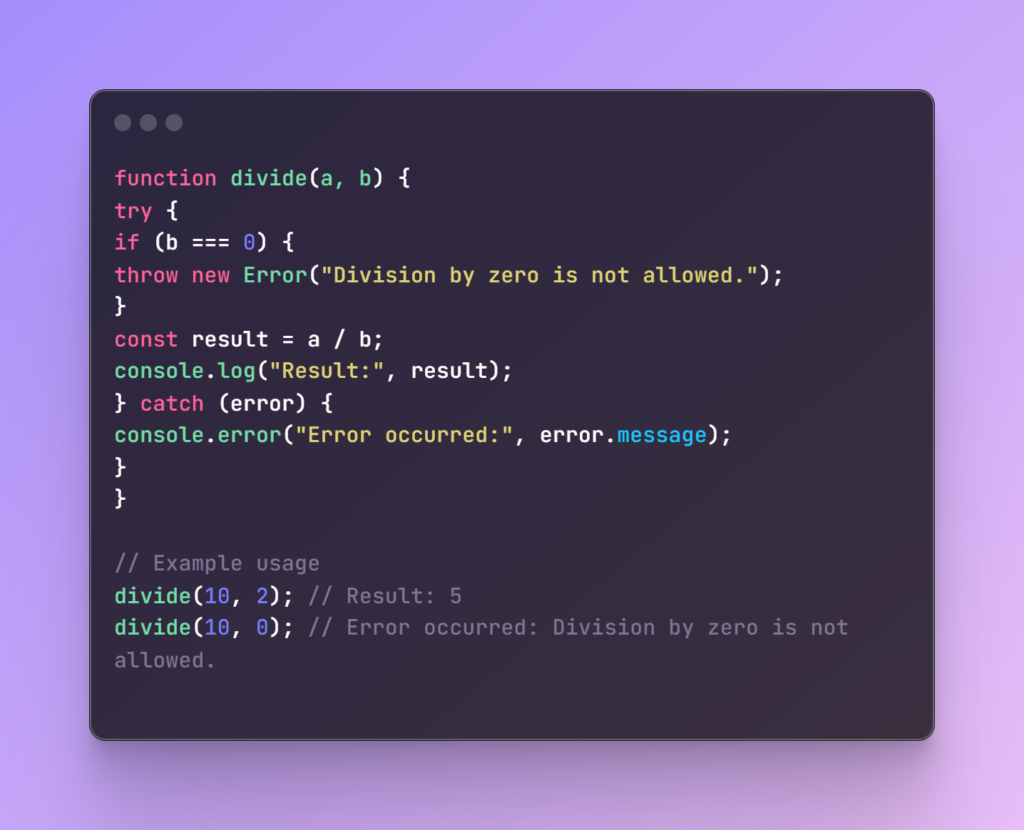
In this example, the divide function attempts to perform a division operation. If the divisor b is zero, it throws a new Error object with a custom error message. The catch block catches the exception, and the error message is logged to the console.
Additionally, you can use the finally block after the catch block, which will always execute regardless of whether an exception occurred or not. This block is optional but useful for cleanup operations.
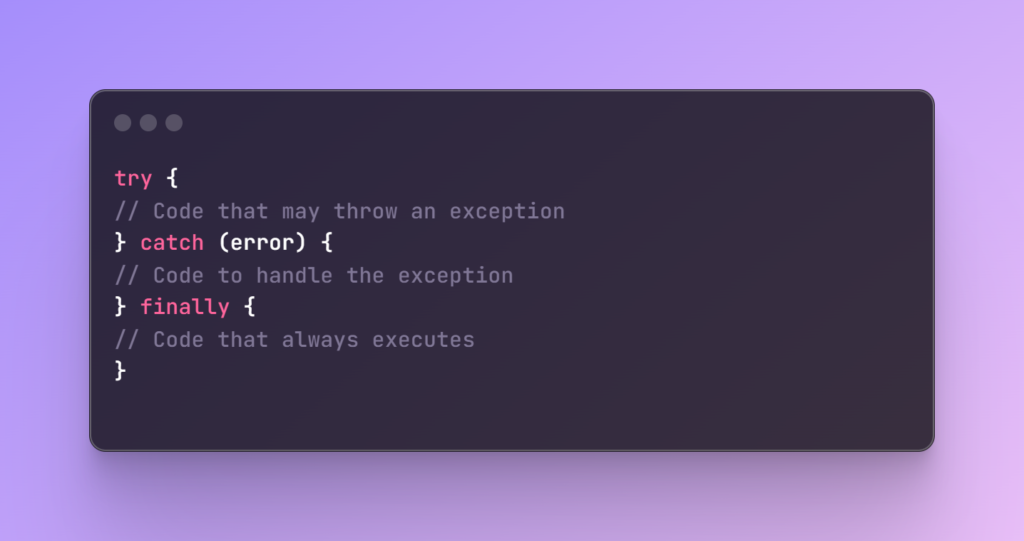
By catching and handling exceptions, you can gracefully handle errors in your JavaScript code and provide appropriate error messages or take alternative actions as needed.
Reference:
javaScript
Read More Blog: