Mastering Advanced JavaScript: Nailing Your Interview with 10 In-Depth Questions
Introduction:
Welcome to our comprehensive guide on mastering advanced JavaScript for your upcoming interview. In this blog post, we’ll explore 10 advanced JavaScript interview questions, diving into the nuances of the language and showcasing your proficiency in its more intricate aspects.
JavaScript Interview Questions Advanced
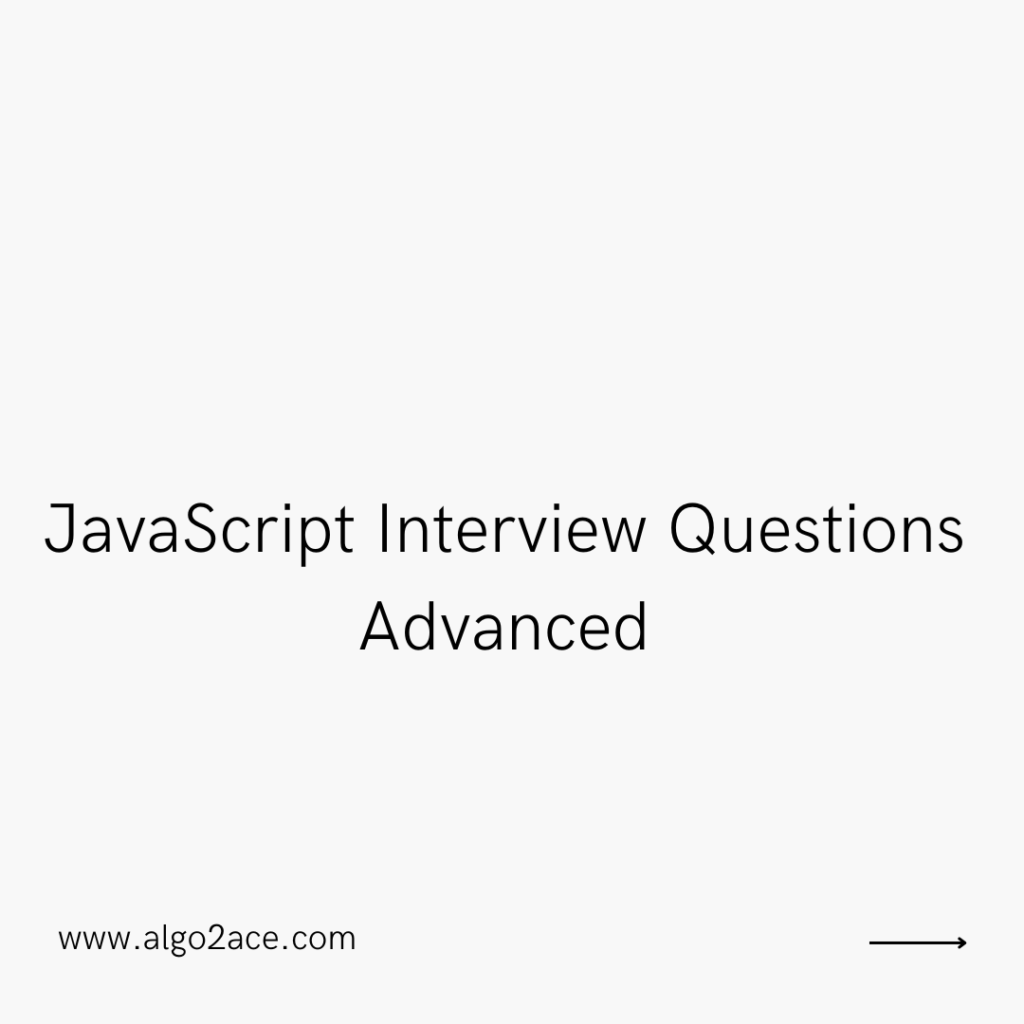
1. Exploring Event Delegation
Explain the concept of event delegation in JavaScript.
Event delegation is a powerful technique that optimizes event handling in the DOM. Rather than attaching an event listener to each individual element, it involves attaching a single event listener to a common ancestor. This leverages the event bubbling phase, enabling efficient event delegation, particularly in scenarios with dynamically added elements.
2. Unveiling the Power of Closures
What is a closure, and how can it be used effectively in JavaScript?
Closures are a fascinating concept in JavaScript, allowing functions to retain access to variables from their outer scopes even after those scopes have completed execution. Understanding closures is crucial for implementing private variables, data encapsulation, and maintaining state in functional programming.
3. Mastering `apply`, `call`, and `bind`
Discuss the differences between the `apply`, `call`, and `bind` methods in JavaScript.
These methods are pivotal for setting the context (`this` value) for a function. While `apply` and `call` are similar, differing in how arguments are passed, `bind` returns a new function with the specified context and arguments without immediate invocation. Understanding these methods enhances your ability to control function contexts in complex scenarios.
4. Demystifying the Event Loop
How does the JavaScript event loop work, and what is the role of the callback queue?
Understanding the event loop is fundamental for handling asynchronous operations in JavaScript. The event loop continuously checks the call stack and the callback queue, facilitating non-blocking asynchronous execution. Insight into this mechanism is vital for building responsive and efficient applications.
5. The Art of Memoization
Explain the concept of memoization and how it can be implemented in JavaScript.
Memoization is a performance optimization technique involving the caching of results from expensive function calls. By storing and returning cached results, developers can significantly improve the performance of functions, particularly those with repetitive or computationally intensive operations.
6. WebSockets vs. Traditional HTTP
What are WebSockets, and how do they differ from traditional HTTP communication?
WebSockets provide a real-time, bidirectional communication channel over a single, long-lived connection. This stands in contrast to traditional HTTP, which follows a request-response model. Understanding the advantages and use cases for WebSockets is crucial for building modern, real-time web applications.
7. The Significance of the `Symbol` Data Type
Describe the role of the `Symbol` data type in JavaScript.
Introduced in ECMAScript 6, `Symbol` is a unique and immutable primitive data type. It is often used as an identifier for object properties, aiding in the creation of non-enumerable properties and helping to avoid naming conflicts in JavaScript code.
8. Harnessing the Power of `Proxy` for Metaprogramming
What is the JavaScript `Proxy` object, and how can it be used for metaprogramming?
The `Proxy` object enables developers to create custom behaviors for fundamental operations on objects, such as property access, assignment, and function invocation. This powerful feature facilitates metaprogramming, allowing the modification of default object behavior for advanced use cases.
9. Exploring Currying and Partial Application
Explain the concepts of currying and partial application in JavaScript.
Currying involves transforming a function with multiple arguments into a series of functions, each taking a single argument. Partial application, on the other hand, entails providing a subset of arguments to a function, producing a new function that takes the remaining arguments. These functional programming techniques enhance code flexibility and reusability.
10. The Role of Service Workers in Web Development
What is the role of a service worker in web development, and how does it enhance web applications?
Service workers are scripts that run in the background, separate from web pages, providing capabilities such as intercepting network requests, caching resources, and enabling features like push notifications and background synchronization. Understanding service workers is crucial for enhancing the performance and offline capabilities of web applications.
Reference:
Read More Blogs:
JavaScript Interview Questions And Answers
Logical Interview Questions In JavaScript
Conclusion:
In conclusion, mastering these advanced JavaScript concepts will not only prepare you for challenging interview questions but also deepen your understanding of the language. Each topic covered in this guide contributes to your ability to write efficient, scalable, and maintainable JavaScript code. Happy coding and good luck with your interview!
Reference