Introduction:
Discover essential Best Dart Interview Questions For Freshers – Prepare Effectively with detailed answers to boost your confidence and performance.
Dart is an open-source, general-purpose programming language developed by Google. It has become highly popular in recent years due to its seamless integration with the Flutter framework, making it an excellent choice for cross-platform mobile app development. Dart’s flexibility, speed, and support for both client-side and server-side development have made it a preferred language for developers aiming to build high-performance mobile, web, and desktop applications.
If you’re a fresher looking to dive into the world of Dart, being well-prepared for an interview is essential. In this guide, we will walk you through some of the most common Dart interview questions that focus on both the foundational concepts of Dart as well as practical aspects, especially its use in Flutter development.
These questions are designed to help freshers demonstrate their understanding of Dart’s syntax, object-oriented features, and key concepts like asynchronous programming and error handling. Let’s explore the questions that can help you ace your Dart interviews as a fresher!
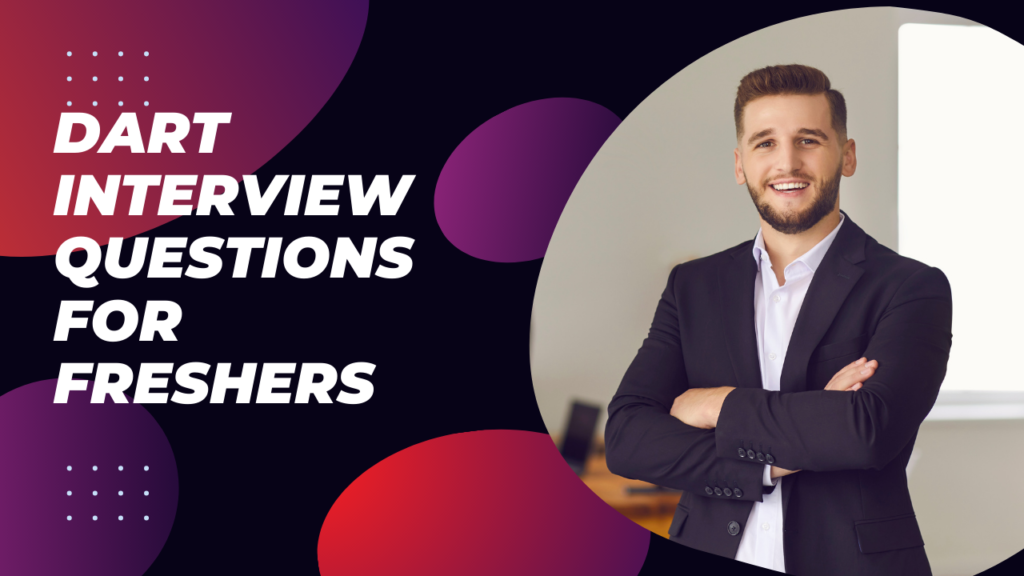
Best Dart Interview Questions for Freshers – Prepare Effectively
1. What is Dart, and why is it used?
Dart is a general-purpose, object-oriented programming language developed by Google. It is used primarily for building mobile, web, and desktop applications. Dart is the primary language for Flutter, which is a UI framework for building cross-platform apps with a single codebase. Its strong performance, simple syntax, and fast development cycle make it ideal for modern app development.
2. What is the difference between var
, final
, and const
in Dart?
var: A variable declared with var can have its value changed after initialization. It is mutable.
var name = 'John';
name = 'Doe'; // This is allowed.
final: A variable declared with final can only be set once and cannot be changed later. It is immutable.
final name = 'John';
name = 'Doe'; // Error: This is not allowed.
const: Similar to final, but const is used for compile-time constants. Variables declared with const must be known at compile time.
const pi = 3.14159; // Compile-time constant.
3. What is a null-aware operator in Dart?
Null-aware operators in Dart help to handle nullable types more easily, without running into null reference errors. Common null-aware operators include:
??: Provides a fallback value if the first expression is null.
var name = null;
print(name ?? 'Anonymous'); // Output: Anonymous
??=: Assigns a value only if the variable is null.
var name;
name ??= 'John';
print(name); // Output: John
4. What is the use of the async
and await
keywords in Dart?
async
and await
are used to handle asynchronous operations in Dart, such as API calls, file I/O, and time-delayed tasks.
async
: Marks a function as asynchronous and returns a Future
.
await: Pauses the execution of an async function until the awaited Future is completed.
Future<void> fetchData() async {
var data = await fetchFromAPI();
print('Data: $data');
}
5. Explain the use of Future
and Stream
in Dart.
Future
and Stream
in Dart.Future: A Future represents a single asynchronous computation that might complete in the future with a value or an error.
Future<String> fetchData() async {
return "Data fetched";
}
Stream
: A Stream
represents a sequence of asynchronous data events. It can emit multiple values over time.
Stream<int> countStream() async* {
for (int i = 1; i <= 5; i++) {
yield i;
await Future.delayed(Duration(seconds: 1));
}
}
6. What is Flutter, and how does Dart relate to it?
Flutter is a UI toolkit developed by Google for building natively compiled applications for mobile, web, and desktop from a single codebase. Dart is the programming language used in Flutter because of its fast development speed and hot reload feature. Dart’s object-oriented nature and easy syntax allow Flutter to create responsive, high-performance UI components for apps.
7. What are mixins in Dart?
A mixin is a way to add functionality to classes in Dart. Mixins allow you to reuse code across different classes without inheritance.
Example:
mixin Swimmable {
void swim() {
print("Swimming...");
}
}
class Fish with Swimmable {}
class Duck with Swimmable {}
// Both Fish and Duck can swim.
8. How do you handle exceptions in Dart?
Exceptions in Dart are handled using try
, catch
, on
, and finally
blocks:
try
: The code that might throw an exception is wrapped in a try
block.
catch
: Used to catch the exception and handle it.
on
: Used to catch specific types of exceptions.
finally
: Code that always runs, regardless of whether an exception occurred.
try {
var result = 10 ~/ 0;
} catch (e) {
print('Error: $e');
} finally {
print('Operation completed.');
}
9. What is the significance of await for
in Dart?
await for
is used to asynchronously iterate over the elements of a stream. It is typically used with Stream
objects when you need to process each item as it arrives.
Stream<int> numberStream() async* {
for (int i = 1; i <= 3; i++) {
yield i;
await Future.delayed(Duration(seconds: 1));
}
}
void printNumbers() async {
await for (var number in numberStream()) {
print(number);
}
}
10. What is the purpose of the late
keyword in Dart?
The late
keyword is used to initialize a non-nullable variable lazily, meaning the variable is initialized when it’s accessed for the first time, rather than at the time of declaration.
late String greeting;
void setGreeting() {
greeting = "Hello, Dart!";
}
Medium-level set of Dart interview questions
1. What are Isolates
in Dart, and why are they important?
Isolates are independent workers that allow parallel execution in Dart. Unlike threads in other languages, isolates don’t share memory; instead, they communicate by passing messages between each other. This prevents issues like race conditions and deadlocks in concurrent programming.
Isolates are essential in Dart, especially for long-running tasks, because Dart’s single-threaded nature can cause the UI to freeze if heavy computations are performed without isolates.
import 'dart:isolate';
void runIsolate(SendPort sendPort) {
sendPort.send('Hello from isolate');
}
void main() async {
ReceivePort receivePort = ReceivePort();
await Isolate.spawn(runIsolate, receivePort.sendPort);
receivePort.listen((message) => print(message)); // Output: Hello from isolate
}
2. What are the different types of constructors in Dart?
Dart offers several types of constructors:
- Default Constructor: A constructor with no parameters.
- Named Constructor: Allows creating multiple constructors for a class using different names.
class Car {
Car(); // Default constructor
Car.sedan(); // Named constructor
}
- Factory Constructor: Used when you want more control over instance creation, often for caching or creating a singleton.
class Logger {
static final Logger _instance = Logger._internal();
factory Logger() => _instance; // Singleton pattern
Logger._internal();
}
3. Explain the concept of closures in Dart.
A closure is a function that can capture variables from its lexical scope, even when the function is called outside that scope. Closures are particularly useful when dealing with callbacks or event handlers.
Function makeAdder(int addBy) {
return (int i) => i + addBy; // `addBy` is captured by the closure
}
void main() {
var add2 = makeAdder(2);
print(add2(3)); // Output: 5
}
4. What is the Cascade Notation
in Dart?
The cascade notation (..
) allows you to make a sequence of operations on the same object. It helps avoid repeated references to the object.
var person = Person()
..name = 'John'
..age = 30
..printInfo();
This is equivalent to:
person.name = 'John';
person.age = 30;
person.printInfo();
5. What is the difference between Stream
and Future
in Dart?
Future
: Represents a single value or error that will be available at some point in the future.
Stream
: Delivers a sequence of values or errors over time. You can subscribe to a stream to handle its events.
Use Future
for one-time events (e.g., fetching data), and Stream
for multiple events (e.g., listening to user input).
Future getNumber() async {
return 42;
}
Stream numberStream() async* {
for (int i = 0; i < 3; i++) {
yield i;
}
}
6. What is the async*
keyword, and when would you use it?
The async*
keyword is used to return a Stream
in Dart. This allows you to yield multiple values asynchronously, typically in situations where you need to generate data over time.
Stream<int> countStream() async* {
for (int i = 1; i <= 5; i++) {
yield i;
await Future.delayed(Duration(seconds: 1)); // Asynchronous yielding
}
}
void main() async {
await for (var value in countStream()) {
print(value); // Output: 1, 2, 3, 4, 5
}
}
7. What are Generics
in Dart, and why are they useful?
Generics allow you to define classes, methods, and functions that can work with any data type. This adds flexibility and reusability to the code while maintaining type safety..
class Box<T> {
T value;
Box(this.value);
}
void main() {
var intBox = Box<int>(42); // Box containing an integer
var stringBox = Box<String>('Hello'); // Box containing a string
}
8. What are extension methods
in Dart?
Extension methods allow you to add new functionality to existing classes without modifying them. This is useful for adding utility functions to standard library classes or your own.
extension StringExtension on String {
String reverse() {
return split('').reversed.join();
}
}
void main() {
print('hello'.reverse()); // Output: olleh
}
9. What is the difference between synchronous and asynchronous generators in Dart?
Synchronous Generator (sync*
): Generates values one at a time and is used in synchronous code.
Iterable syncGenerator() sync* {
yield 1;
yield 2;
}
Asynchronous Generator (async*
): Generates values asynchronously over time and returns a Stream
.
Stream asyncGenerator() async* {
await Future.delayed(Duration(seconds: 1));
yield 1;
}
10. Explain the use of Zone
in Dart.
A Zone
in Dart provides a mechanism to handle asynchronous code in a controlled environment. It allows you to intercept and manage errors, timers, and other asynchronous events. Zones are useful for tracking operations in testing or modifying behavior without touching the original code.
void main() {
runZonedGuarded(() {
Future.delayed(Duration(seconds: 1), () {
throw 'An error occurred!';
});
}, (error, stack) {
print('Caught error: $error');
});
}
Reference :
React Scenario Based Interview Questions[Answered]
Best Java Scenario Based Interview Questions[Answered]
Conclusion:
Conclusion:
Mastering Dart is essential for developers looking to build modern cross-platform applications, especially with the rise of frameworks like Flutter. By understanding concepts such as asynchronous programming, isolates, streams, and language-specific features like mixins and extension methods, you can effectively utilize Dart’s capabilities in both small and large-scale applications.
Whether you’re preparing for an interview or enhancing your programming skills, focusing on the key areas covered in these questions will give you a solid foundation in Dart. From basic syntax to advanced concepts like async*
, await
, and isolates, a well-rounded knowledge of Dart ensures you are ready to tackle both practical coding challenges and theoretical questions in your interviews.