Introduction:
Welcome to the new post JavaScript Interview Questions For 5 Years Experience
In the ever-evolving landscape of web development, JavaScript stands as the cornerstone of interactive and dynamic user experiences. For seasoned developers with approximately five years of experience, JavaScript knowledge is not just a skill but a testament to their journey in the realm of programming. As interviews become gateways to new opportunities, let’s delve into 15 JavaScript interview questions tailored for those with substantial hands-on experience. Whether you’re gearing up for a job change or aiming to solidify your expertise, these questions will serve as a compass to navigate the intricacies of JavaScript.
JavaScript Interview Questions For 5 Years Experience
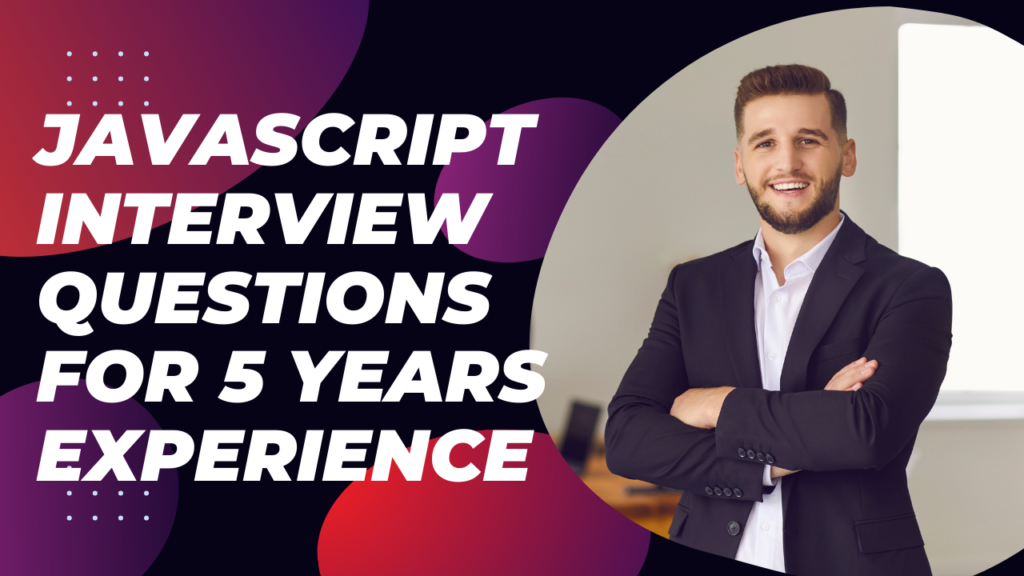
1. Explain the concept of “hoisting” in JavaScript.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase. This allows variables and functions to be used before they are declared.
2. How does ES6 arrow function differ from traditional function expressions?
Arrow functions have a more concise syntax, do not have their own `this` binding (inherits from the enclosing scope), and do not have the `arguments` object.
3. Describe the role of closures in JavaScript and provide an example.
Closures allow functions to retain access to variables from their lexical scope, even after the outer function has finished execution. Example:
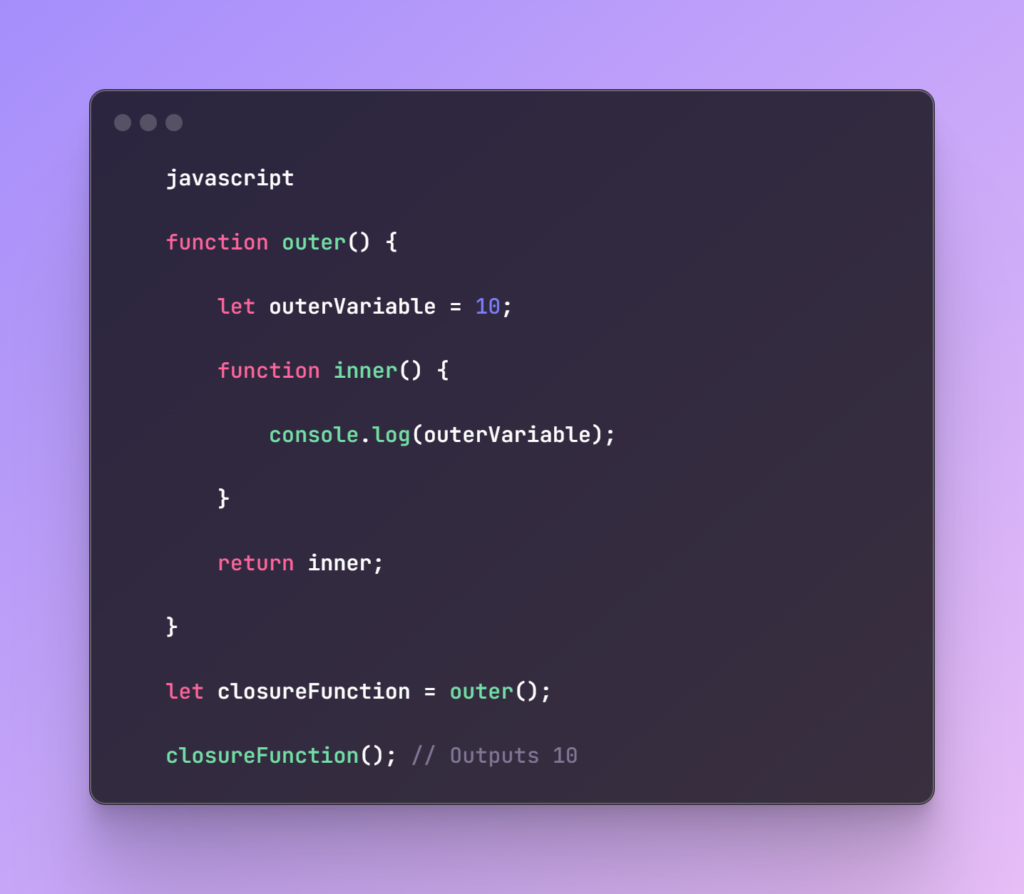
4. What is the event loop in JavaScript, and how does it work?
The event loop is a mechanism that allows JavaScript to handle asynchronous operations. It consists of a call stack, callback queue, and event loop. It continuously checks the callback queue and pushes callbacks onto the call stack when the stack is empty.
5. Explain the concept of Promises and how they help manage asynchronous operations.
Promises are objects representing the eventual completion or failure of an asynchronous operation. They have states (pending, fulfilled, rejected) and allow chaining of multiple asynchronous operations, making it easier to handle asynchronous code.
6. How does prototypal inheritance work in JavaScript?
Prototypal inheritance is a mechanism where objects can inherit properties and methods from other objects.
Each object has a prototype, and properties/methods not found in the object itself are looked up in its prototype chain.
7. Differentiate between `let`, `const`, and `var` in terms of variable declaration.
`let` and `const` were introduced in ES6. `let` allows reassignment of values, while `const` is used for variables with constant values. `var` is function-scoped and can be reassigned.
8. How does the `async/await` syntax simplify working with asynchronous code?
`async/await` is a syntax for handling asynchronous code that makes it look and behave more like synchronous code.
It allows the use of `await` to pause the execution of the function until the promise is resolved.
9. What is a RESTful API, and how can it be implemented in JavaScript?
RESTful APIs follow the principles of Representational State Transfer. In JavaScript, you can use frameworks like Express.js to implement RESTful APIs, defining routes and handling HTTP methods.
10. Explain the concept of debouncing and provide a use case.
Debouncing is a technique to ensure that time-consuming tasks do not fire so often, making them more efficient.
It is often used in scenarios like handling search input where you want to wait for the user to finish typing before triggering a search.
11. What are the differences between `localStorage` and `sessionStorage`?
Both `localStorage` and `sessionStorage` are web storage objects, but `localStorage` persists even after the browser is closed, while `sessionStorage` is limited to a session.
12. Discuss the purpose of the `map()` function in JavaScript.
The `map()` function creates a new array by applying a provided function to each element of the array. It does not modify the original array.
13. How can you handle cross-origin requests in JavaScript?
Cross-origin requests can be handled using techniques like JSONP, CORS (Cross-Origin Resource Sharing), or by using server-side proxies.
14. Explain the concept of a callback function and provide an example.
A callback function is a function passed as an argument to another function, to be executed later. Example:
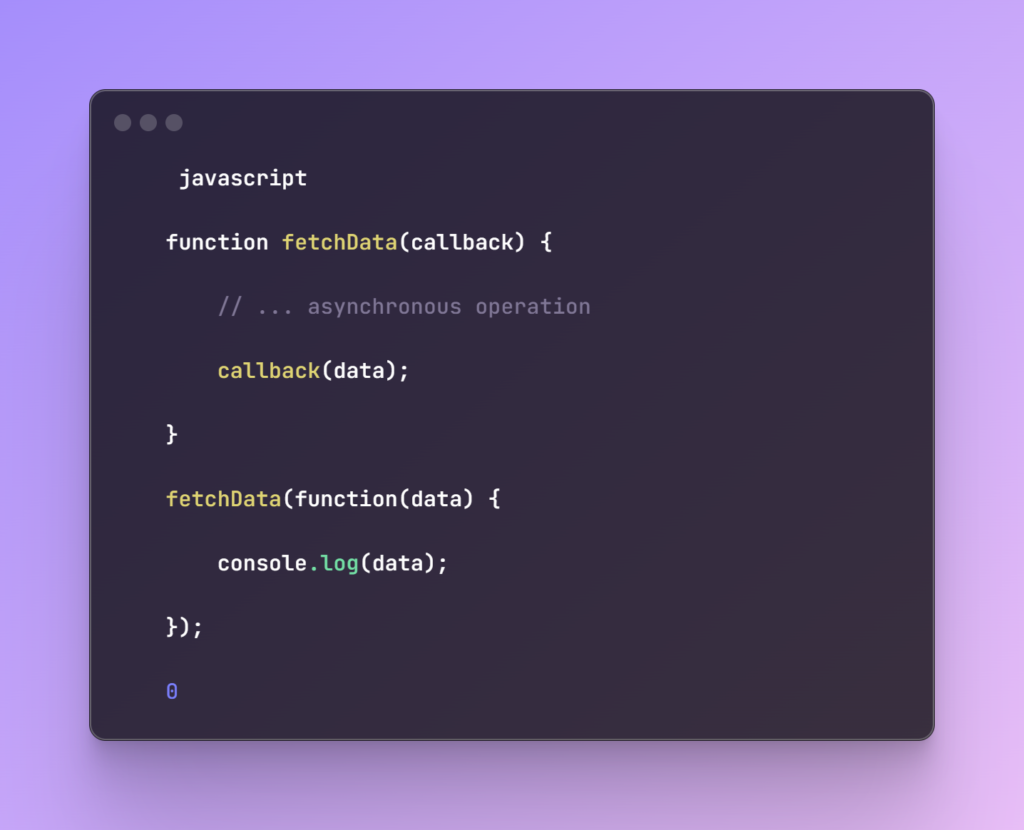
15. Discuss the role of the `this` keyword in JavaScript and how it can be controlled.
`this` refers to the current execution context. In JavaScript, its value is determined by how a function is called. The `bind()`, `call()`, and `apply()` methods can be used to control the value of `this` in a function.
Reference:
Javascript Scenario-Based Interview Questions[Answered]
Conclusion:
Mastering JavaScript is a journey marked by continuous learning and adaptation. As you prepare for interviews or seek to enhance your proficiency, these 15 questions provide a comprehensive roadmap. With a solid foundation in hoisting, closures, prototypes, and the nuances of modern JavaScript, you’re well-equipped to showcase your expertise and elevate your career. Embrace the challenges, stay curious, and let your journey through JavaScript continue to unfold.